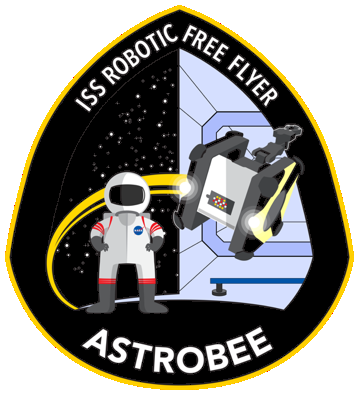 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
39 #ifndef PCL_REGISTRATION_CORRESPONDENCE_REJECTION_SURFACE_NORMAL2_H_ // NOLINT
40 #define PCL_REGISTRATION_CORRESPONDENCE_REJECTION_SURFACE_NORMAL2_H_ // NOLINT
42 #include <pcl/registration/correspondence_rejection.h>
43 #include <pcl/point_cloud.h>
46 namespace registration {
62 template <
typename Po
intWithNormalT>
64 using CorrespondenceRejector::getClassName;
65 using CorrespondenceRejector::input_correspondences_;
66 using CorrespondenceRejector::rejection_name_;
69 typedef boost::shared_ptr<CorrespondenceRejectorSurfaceNormal2>
Ptr;
70 typedef boost::shared_ptr<const CorrespondenceRejectorSurfaceNormal2>
ConstPtr;
74 rejection_name_ =
"CorrespondenceRejectorSurfaceNormal2";
88 inline void setThreshold(
double threshold) { threshold_ = threshold; }
94 pcl::Correspondences& remaining_correspondences) {
95 if (!data_container_) {
96 PCL_ERROR(
"[pcl::registratin::%s::getRemainingCorrespondences] DataContainer object is not initialized!\n",
97 getClassName().c_str());
101 unsigned int number_valid_correspondences = 0;
102 remaining_correspondences.resize(original_correspondences.size());
105 for (
size_t i = 0; i < original_correspondences.size(); ++i) {
107 if (std::abs(boost::static_pointer_cast<DataContainer<PointWithNormalT, PointWithNormalT> >(data_container_)
108 ->getCorrespondenceScoreFromNormals(original_correspondences[i])) > threshold_) {
109 remaining_correspondences[number_valid_correspondences++] = original_correspondences[i];
112 remaining_correspondences.resize(number_valid_correspondences);
117 data_container_.reset(
new DataContainer<PointWithNormalT, PointWithNormalT>);
123 inline void setInputCloud(
const typename pcl::PointCloud<PointWithNormalT>::ConstPtr& input) {
124 PCL_WARN(
"[pcl::registration::%s::setInputCloud] setInputCloud is deprecated. Please use setInputSource instead.\n",
125 getClassName().c_str());
126 if (!data_container_) {
128 "[pcl::registration::%s::setInputCloud] Initialize the data container object by calling intializeDataContainer "
129 "() before using this function.\n",
130 getClassName().c_str());
133 boost::static_pointer_cast<DataContainer<PointWithNormalT> >(data_container_)->setInputSource(input);
139 inline void setInputSource(
const typename pcl::PointCloud<PointWithNormalT>::ConstPtr& input) {
140 if (!data_container_) {
142 "[pcl::registration::%s::setInputCloud] Initialize the data container object by calling intializeDataContainer "
143 "() before using this function.\n",
144 getClassName().c_str());
147 boost::static_pointer_cast<DataContainer<PointWithNormalT> >(data_container_)->setInputSource(input);
151 inline typename pcl::PointCloud<PointWithNormalT>::ConstPtr
getInputSource()
const {
152 if (!data_container_) {
154 "[pcl::registration::%s::getInputSource] Initialize the data container object by calling "
155 "intializeDataContainer () before using this function.\n",
156 getClassName().c_str());
159 return (boost::static_pointer_cast<DataContainer<PointWithNormalT> >(data_container_)->getInputSource());
165 inline void setInputTarget(
const typename pcl::PointCloud<PointWithNormalT>::ConstPtr& target) {
166 if (!data_container_) {
168 "[pcl::registration::%s::setInputTarget] Initialize the data container object by calling "
169 "intializeDataContainer () before using this function.\n",
170 getClassName().c_str());
173 boost::static_pointer_cast<DataContainer<PointWithNormalT> >(data_container_)->setInputTarget(target);
184 bool force_no_recompute =
false) {
185 boost::static_pointer_cast<DataContainer<PointWithNormalT> >(data_container_)
186 ->setSearchMethodTarget(tree, force_no_recompute);
190 inline typename pcl::PointCloud<PointWithNormalT>::ConstPtr
getInputTarget()
const {
191 if (!data_container_) {
193 "[pcl::registration::%s::getInputTarget] Initialize the data container object by calling "
194 "intializeDataContainer () before using this function.\n",
195 getClassName().c_str());
198 return (boost::static_pointer_cast<DataContainer<PointWithNormalT> >(data_container_)->getInputTarget());
204 inline void setInputNormals(
const typename pcl::PointCloud<PointWithNormalT>::ConstPtr& normals) {
205 if (!data_container_) {
207 "[pcl::registration::%s::setInputNormals] Initialize the data container object by calling "
208 "intializeDataContainer () before using this function.\n",
209 getClassName().c_str());
212 boost::static_pointer_cast<DataContainer<PointWithNormalT, PointWithNormalT> >(data_container_)
213 ->setInputNormals(normals);
218 if (!data_container_) {
220 "[pcl::registration::%s::getInputNormals] Initialize the data container object by calling "
221 "intializeDataContainer () before using this function.\n",
222 getClassName().c_str());
225 return (boost::static_pointer_cast<DataContainer<PointWithNormalT, PointWithNormalT> >(data_container_)
226 ->getInputNormals());
232 inline void setTargetNormals(
const typename pcl::PointCloud<PointWithNormalT>::ConstPtr& normals) {
233 if (!data_container_) {
235 "[pcl::registration::%s::setTargetNormals] Initialize the data container object by calling "
236 "intializeDataContainer () before using this function.\n",
237 getClassName().c_str());
240 boost::static_pointer_cast<DataContainer<PointWithNormalT, PointWithNormalT> >(data_container_)
241 ->setTargetNormals(normals);
246 if (!data_container_) {
248 "[pcl::registration::%s::getTargetNormals] Initialize the data container object by calling "
249 "intializeDataContainer () before using this function.\n",
250 getClassName().c_str());
253 return (boost::static_pointer_cast<DataContainer<PointWithNormalT, PointWithNormalT> >(data_container_)
254 ->getTargetNormals());
262 if (!data_container_) initializeDataContainer();
263 typename PointCloud<PointWithNormalT>::Ptr cloud(
new PointCloud<PointWithNormalT>);
264 fromPCLPointCloud2(*cloud2, *cloud);
265 setInputSource(cloud);
273 if (!data_container_) initializeDataContainer();
274 typename PointCloud<PointWithNormalT>::Ptr cloud(
new PointCloud<PointWithNormalT>);
275 fromPCLPointCloud2(*cloud2, *cloud);
276 setInputTarget(cloud);
284 if (!data_container_) initializeDataContainer();
285 typename PointCloud<PointWithNormalT>::Ptr cloud(
new PointCloud<PointWithNormalT>);
286 fromPCLPointCloud2(*cloud2, *cloud);
287 setInputNormals(cloud);
295 if (!data_container_) initializeDataContainer();
296 typename PointCloud<PointWithNormalT>::Ptr cloud(
new PointCloud<PointWithNormalT>);
297 fromPCLPointCloud2(*cloud2, *cloud);
298 setTargetNormals(cloud);
306 getRemainingCorrespondences(*input_correspondences_, correspondences);
Definition: correspondence_rejection_surface_normal2.h:45
void setInputNormals(const typename pcl::PointCloud< PointWithNormalT >::ConstPtr &normals)
Set the normals computed on the input point cloud.
Definition: correspondence_rejection_surface_normal2.h:204
pcl::PointCloud< PointWithNormalT >::ConstPtr getInputTarget() const
Get the target input point cloud.
Definition: correspondence_rejection_surface_normal2.h:190
void setTargetPoints(pcl::PCLPointCloud2::ConstPtr cloud2)
Method for setting the target cloud.
Definition: correspondence_rejection_surface_normal2.h:272
boost::shared_ptr< DataContainerInterface > DataContainerPtr
Definition: correspondence_rejection_surface_normal2.h:312
boost::shared_ptr< CorrespondenceRejectorSurfaceNormal2 > Ptr
Definition: correspondence_rejection_surface_normal2.h:69
pcl::PointCloud< PointWithNormalT >::Ptr getInputNormals() const
Get the normals computed on the input point cloud.
Definition: correspondence_rejection_surface_normal2.h:217
void setInputCloud(const typename pcl::PointCloud< PointWithNormalT >::ConstPtr &input)
Provide a source point cloud dataset (must contain XYZ data!), used to compute the correspondence dis...
Definition: correspondence_rejection_surface_normal2.h:123
void setTargetNormals(pcl::PCLPointCloud2::ConstPtr cloud2)
Method for setting the target normals.
Definition: correspondence_rejection_surface_normal2.h:294
void setInputTarget(const typename pcl::PointCloud< PointWithNormalT >::ConstPtr &target)
Provide a target point cloud dataset (must contain XYZ data!), used to compute the correspondence dis...
Definition: correspondence_rejection_surface_normal2.h:165
void applyRejection(pcl::Correspondences &correspondences)
Apply the rejection algorithm.
Definition: correspondence_rejection_surface_normal2.h:305
void getRemainingCorrespondences(const pcl::Correspondences &original_correspondences, pcl::Correspondences &remaining_correspondences)
Definition: correspondence_rejection_surface_normal2.h:93
void setSearchMethodTarget(const boost::shared_ptr< pcl::search::KdTree< PointWithNormalT > > &tree, bool force_no_recompute=false)
Provide a pointer to the search object used to find correspondences in the target cloud.
Definition: correspondence_rejection_surface_normal2.h:183
void setSourceNormals(pcl::PCLPointCloud2::ConstPtr cloud2)
Blob method for setting the source normals.
Definition: correspondence_rejection_surface_normal2.h:283
pcl::PointCloud< PointWithNormalT >::Ptr getTargetNormals() const
Get the normals computed on the target point cloud.
Definition: correspondence_rejection_surface_normal2.h:245
void setSourcePoints(pcl::PCLPointCloud2::ConstPtr cloud2)
Blob method for setting the source cloud.
Definition: correspondence_rejection_surface_normal2.h:261
DataContainerPtr data_container_
A pointer to the DataContainer object containing the input and target point clouds.
Definition: correspondence_rejection_surface_normal2.h:314
void setThreshold(double threshold)
Get a list of valid correspondences after rejection from the original set of correspondences.
Definition: correspondence_rejection_surface_normal2.h:88
bool requiresTargetPoints() const
See if this rejector requires a target cloud.
Definition: correspondence_rejection_surface_normal2.h:269
void initializeDataContainer()
Initialize the data container object for the point type and the normal type.
Definition: correspondence_rejection_surface_normal2.h:116
double threshold_
The median distance threshold between two correspondent points in source <-> target.
Definition: correspondence_rejection_surface_normal2.h:310
double getThreshold() const
Get the thresholding angle between the normals for correspondence rejection.
Definition: correspondence_rejection_surface_normal2.h:91
void setTargetNormals(const typename pcl::PointCloud< PointWithNormalT >::ConstPtr &normals)
Set the normals computed on the target point cloud.
Definition: correspondence_rejection_surface_normal2.h:232
bool requiresSourceNormals() const
See if this rejector requires source normals.
Definition: correspondence_rejection_surface_normal2.h:280
bool requiresTargetNormals() const
See if this rejector requires target normals.
Definition: correspondence_rejection_surface_normal2.h:291
CorrespondenceRejectorSurfaceNormal2()
Empty constructor. Sets the threshold to 1.0.
Definition: correspondence_rejection_surface_normal2.h:73
pcl::PointCloud< PointWithNormalT >::ConstPtr getInputSource() const
Get the target input point cloud.
Definition: correspondence_rejection_surface_normal2.h:151
Definition: correspondence_rejection_surface_normal2.h:63
void setInputSource(const typename pcl::PointCloud< PointWithNormalT >::ConstPtr &input)
Provide a source point cloud dataset (must contain XYZ data!), used to compute the correspondence dis...
Definition: correspondence_rejection_surface_normal2.h:139
boost::shared_ptr< const CorrespondenceRejectorSurfaceNormal2 > ConstPtr
Definition: correspondence_rejection_surface_normal2.h:70
bool requiresSourcePoints() const
See if this rejector requires source points.
Definition: correspondence_rejection_surface_normal2.h:258