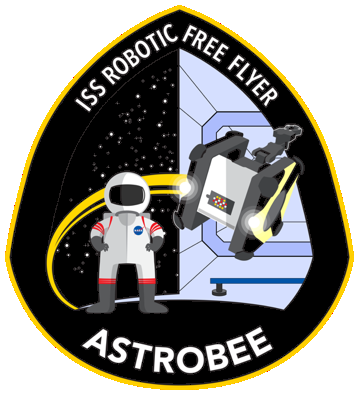 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_PRO_CONVERSIONS_H_
20 #define TRAJ_OPT_PRO_CONVERSIONS_H_
22 #include <geometry_msgs/Point.h>
23 #include <geometry_msgs/Vector3.h>
32 v << p.x, p.y, p.z, 0.0;
36 geometry_msgs::Point p;
44 v << p.x, p.y, p.z, 0.0;
48 geometry_msgs::Vector3 p;
56 #endif // TRAJ_OPT_PRO_CONVERSIONS_H_
Definition: conversions.h:27
static Vec4 PointToVec(const geometry_msgs::Point &p)
Definition: conversions.h:30
static geometry_msgs::Point VecToPoint(const Vec4 &v)
Definition: conversions.h:35
Definition: msg_traj.h:27
Eigen::Matrix< decimal_t, 4, 1 > Vec4
Definition: types.h:41
static Vec4 Vector3ToVec(const geometry_msgs::Vector3 &p)
Definition: conversions.h:42
static geometry_msgs::Vector3 VecToVector3(const Vec4 &v)
Definition: conversions.h:47