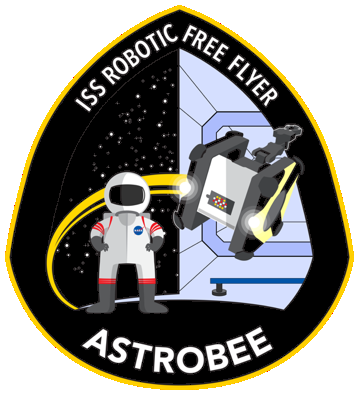 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
20 #ifndef JSONLOADER_COMMAND_REPO_H_
21 #define JSONLOADER_COMMAND_REPO_H_
109 float pan()
const noexcept;
110 float tilt()
const noexcept;
111 std::string
const&
which()
const noexcept;
123 bool open()
const noexcept;
135 std::string
const&
which()
const noexcept;
136 int id()
const noexcept;
146 std::string
const&
apk()
const noexcept;
156 std::string
const&
apk()
const noexcept;
157 std::string
const&
command()
const noexcept;
161 std::string command_;
168 std::string
const&
which()
const noexcept;
185 std::string
const&
camera()
const noexcept;
195 std::string
const&
mode()
const noexcept;
198 std::string
const&
resolution()
const noexcept;
204 std::string resolution_;
211 bool record()
const noexcept;
221 bool stream()
const noexcept;
239 bool checkObstacles_;
259 bool enableHolonomic_;
266 std::string
const&
planner()
const noexcept;
269 std::string planner_;
277 float rate()
const noexcept;
280 std::string telemetryName_;
288 std::string
const&
mode()
const noexcept;
301 std::string description_;
316 #endif // JSONLOADER_COMMAND_REPO_H_
int berth_number() const noexcept
Definition: command_repo.cc:212
EnableAstrobeeIntercommsCommand(Json::Value const &obj)
Definition: command_repo.cc:578
SetCheckObstaclesCommand(Json::Value const &obj)
Definition: command_repo.cc:456
constexpr char kCmdStopGuest[]
Definition: command_repo.h:43
Definition: command_repo.h:294
std::string const & which() const noexcept
Definition: command_repo.cc:360
constexpr char kCmdPowerOff[]
Definition: command_repo.h:41
StopRecordingCommand(Json::Value const &obj)
Definition: command_repo.cc:573
constexpr char kCmdChkObstacles[]
Definition: command_repo.h:53
constexpr char kCmdIdleProp[]
Definition: command_repo.h:48
constexpr char kCmdRecordCamera[]
Definition: command_repo.h:51
Definition: command_repo.h:63
float brightness() const noexcept
Definition: command_repo.cc:364
std::string const & which() const noexcept
Definition: command_repo.cc:306
Definition: command_repo.h:176
PerchCommand(Json::Value const &obj)
Definition: command_repo.cc:221
constexpr char kCmdPause[]
Definition: command_repo.h:34
constexpr char kCmdSetHolonomic[]
Definition: command_repo.h:55
GripperCommand(Json::Value const &obj)
Definition: command_repo.cc:262
bool enableHolonomic() const noexcept
Definition: command_repo.cc:500
DockCommand(Json::Value const &obj)
Definition: command_repo.cc:200
constexpr char kCmdGuestCmd[]
Definition: command_repo.h:44
Definition: command_repo.h:284
Definition: command_repo.h:83
constexpr char kCmdStopRecord[]
Definition: command_repo.h:60
Definition: command_repo.h:131
constexpr char kCmdChkZones[]
Definition: command_repo.h:54
constexpr char kCmdStationKeep[]
Definition: command_repo.h:36
Definition: command_repo.h:262
StationKeepCommand(Json::Value const &obj)
Definition: command_repo.cc:278
constexpr char kCmdSwitchLocal[]
Definition: command_repo.h:58
StartRecordingCommand(Json::Value const &obj)
Definition: command_repo.cc:557
constexpr char kCmdUndock[]
Definition: command_repo.h:31
bool stream() const noexcept
Definition: command_repo.cc:447
Definition: command_repo.h:88
constexpr char kCmdSetPlanner[]
Definition: command_repo.h:56
PowerItemCommand(Json::Value const &obj)
Definition: command_repo.cc:294
Definition: command_repo.h:272
float frame_rate() const noexcept
Definition: command_repo.cc:407
SetPlannerCommand(Json::Value const &obj)
Definition: command_repo.cc:504
constexpr char kCmdCustomGuest[]
Definition: command_repo.h:45
constexpr char kCmdPerch[]
Definition: command_repo.h:32
std::string const & telemetryName() const noexcept
Definition: command_repo.cc:533
FlashlightCommand(Json::Value const &obj)
Definition: command_repo.cc:347
Definition: command_repo.h:242
RecordCameraCommand(Json::Value const &obj)
Definition: command_repo.cc:419
std::string const & apk() const noexcept
Definition: command_repo.cc:322
constexpr char kCmdInitBias[]
Definition: command_repo.h:52
constexpr char kCmdTelemRate[]
Definition: command_repo.h:57
Value
Definition: GPIO.h:42
SetCameraCommand(Json::Value const &obj)
Definition: command_repo.cc:387
std::string const & mode() const noexcept
Definition: command_repo.cc:553
float rate() const noexcept
Definition: command_repo.cc:537
PauseCommand(Json::Value const &obj)
Definition: command_repo.cc:231
Definition: command_repo.h:252
Definition: command_repo.h:304
GuestScienceCommand(Json::Value const &obj)
Definition: command_repo.cc:310
CustomGuestScienceCommand(Json::Value const &obj)
Definition: command_repo.cc:326
constexpr char kCmdFlashlight[]
Definition: command_repo.h:47
constexpr char kCmdPayloadOn[]
Definition: command_repo.h:38
Definition: command_repo.h:105
Definition: command_repo.h:164
Definition: command_repo.h:309
std::string const & planner() const noexcept
Definition: command_repo.cc:516
float tilt() const noexcept
Definition: command_repo.cc:254
SetHolonomicModeCommand(Json::Value const &obj)
Definition: command_repo.cc:488
UndockCommand(Json::Value const &obj)
Definition: command_repo.cc:216
std::string const & resolution() const noexcept
Definition: command_repo.cc:415
Definition: command_repo.h:207
Definition: command_repo.h:191
std::string const & mode() const noexcept
Definition: command_repo.cc:403
constexpr char kCmdStartRecord[]
Definition: command_repo.h:59
bool record() const noexcept
Definition: command_repo.cc:431
Definition: command_repo.h:232
constexpr char kCmdArmPanTilt[]
Definition: command_repo.h:35
ArmPanAndTiltCommand(Json::Value const &obj)
Definition: command_repo.cc:236
std::string const & command() const noexcept
Definition: command_repo.cc:343
constexpr char kCmdPayloadOff[]
Definition: command_repo.h:40
Definition: command_repo.h:152
std::string const & apk() const noexcept
Definition: command_repo.cc:339
Definition: command_repo.h:142
constexpr char kCmdDock[]
Definition: command_repo.h:30
Definition: command_repo.h:119
constexpr char kCmdGripper[]
Definition: command_repo.h:46
std::string const & camera() const noexcept
Definition: command_repo.cc:383
IdlePropulsionCommand(Json::Value const &obj)
Definition: command_repo.cc:368
Definition: command_repo.h:181
constexpr char kCmdStartGuest[]
Definition: command_repo.h:42
constexpr char kCmdSetCamera[]
Definition: command_repo.h:49
SetTelemetryRateCommand(Json::Value const &obj)
Definition: command_repo.cc:520
float duration() const noexcept
Definition: command_repo.cc:290
bool checkZones() const noexcept
Definition: command_repo.cc:484
constexpr char kCmdWait[]
Definition: command_repo.h:37
bool open() const noexcept
Definition: command_repo.cc:274
constexpr char kCmdUnperch[]
Definition: command_repo.h:33
float pan() const noexcept
Definition: command_repo.cc:250
float bandwidth() const noexcept
Definition: command_repo.cc:411
Definition: command_repo.h:95
InitializeBiasCommand(Json::Value const &obj)
Definition: command_repo.cc:451
std::string const & description() const noexcept
Definition: command_repo.cc:569
bool checkObstacles() const noexcept
Definition: command_repo.cc:468
SwitchLocalizationCommand(Json::Value const &obj)
Definition: command_repo.cc:541
std::string const & which() const noexcept
Definition: command_repo.cc:258
Definition: command_repo.h:73
constexpr char kCmdStreamCamera[]
Definition: command_repo.h:50
CameraCommand(Json::Value const &obj)
Definition: command_repo.cc:373
Definition: command_repo.h:227
Definition: command_repo.h:217
Definition: command_repo.h:78
UnperchCommand(Json::Value const &obj)
Definition: command_repo.cc:226
constexpr char kCmdPowerOn[]
Definition: command_repo.h:39
StreamCameraCommand(Json::Value const &obj)
Definition: command_repo.cc:435
SetCheckZonesCommand(Json::Value const &obj)
Definition: command_repo.cc:472
constexpr char kCmdEnableAstrobeeIntercomms[]
Definition: command_repo.h:61