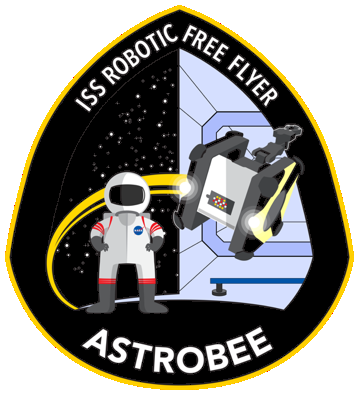 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef JSONLOADER_COMMAND_H_
20 #define JSONLOADER_COMMAND_H_
34 virtual bool valid()
const noexcept;
36 std::string
const&
type()
const noexcept;
63 #endif // JSONLOADER_COMMAND_H_
static Command * Make(Json::Value const &obj)
Definition: command.cc:49
void set_valid(const bool valid) noexcept
Definition: command.cc:107
virtual bool valid() const noexcept
Definition: command.cc:95
Value
Definition: GPIO.h:42
std::string const & type() const noexcept
Definition: command.cc:103
virtual ~Command()
Definition: command.cc:92
jsonloader::Command *(*)(Json::Value const &obj) CommandCreateFn
Definition: command.h:59
jsonloader::Command * CreateCommand(Json::Value const &obj)
Definition: command.h:55
bool blocking() const noexcept
Definition: command.cc:99
Command(Json::Value const &obj)
Definition: command.cc:84