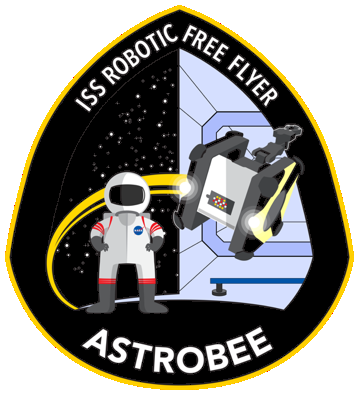 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef IMU_INTEGRATION_BUTTERO7_H_
20 #define IMU_INTEGRATION_BUTTERO7_H_
29 template <
class Params>
35 if (!initialized_) Initialize(value, Params::kGain);
37 const int last_index = xv_.size() - 1;
39 for (
int i = 0; i < last_index; ++i) {
44 xv_[last_index] = value / Params::kGain;
46 yv_[last_index] = (xv_[0] + xv_[9]) + Params::kX18 * (xv_[1] + xv_[8]) + Params::kX27 * (xv_[2] + xv_[7]) +
47 Params::kX36 * (xv_[3] + xv_[6]) + Params::kX45 * (xv_[4] + xv_[5]) + (Params::kY2 * yv_[2]) +
48 (Params::kY3 * yv_[3]) + (Params::kY4 * yv_[4]) + (Params::kY5 * yv_[5]) +
49 (Params::kY6 * yv_[6]) + (Params::kY7 * yv_[7]) + (Params::kY8 * yv_[8]);
52 return yv_[last_index];
56 void Initialize(
const double first_value,
const double gain) {
57 for (
auto& val : xv_) {
58 val = first_value / gain;
60 for (
auto& val : yv_) {
69 std::array<double, 10> xv_;
70 std::array<double, 10> yv_;
75 static constexpr
double kGain = 3.168744781e+06;
76 static constexpr
double kX18 = 7.9994195281;
77 static constexpr
double kX27 = 28.9959366960;
78 static constexpr
double kX36 = 62.9878100890;
79 static constexpr
double kX45 = 90.9796834820;
80 static constexpr
double kY2 = 0.2561484929;
81 static constexpr
double kY3 = -2.1400274434;
82 static constexpr
double kY4 = 7.7016541929;
83 static constexpr
double kY5 = -15.4832538240;
84 static constexpr
double kY6 = 18.7878862180;
85 static constexpr
double kY7 = -13.7678927060;
86 static constexpr
double kY8 = 5.6453639087;
91 #endif // IMU_INTEGRATION_BUTTERO7_H_
double AddValue(const double value) final
Definition: butterO7.h:34
static constexpr double kY4
Definition: butterO7.h:82
static constexpr double kX45
Definition: butterO7.h:79
Definition: butterO1.h:26
static constexpr double kX36
Definition: butterO7.h:78
static constexpr double kY7
Definition: butterO7.h:85
static constexpr double kY3
Definition: butterO7.h:81
static constexpr double kX27
Definition: butterO7.h:77
Definition: butterO7.h:74
static constexpr double kX18
Definition: butterO7.h:76
static constexpr double kGain
Definition: butterO7.h:75
ButterO7()
Definition: butterO7.h:32
Definition: butterO7.h:30
static constexpr double kY8
Definition: butterO7.h:86
static constexpr double kY2
Definition: butterO7.h:80
static constexpr double kY5
Definition: butterO7.h:83
static constexpr double kY6
Definition: butterO7.h:84