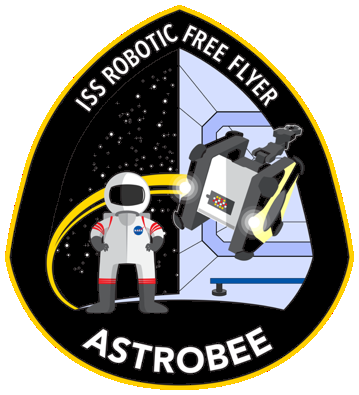 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef EXECUTIVE_EXECUTIVE_H_
20 #define EXECUTIVE_EXECUTIVE_H_
26 #include <ff_hw_msgs/ClearTerminate.h>
27 #include <ff_hw_msgs/ConfigurePayloadPower.h>
28 #include <ff_hw_msgs/ConfigureSystemLeds.h>
29 #include <ff_hw_msgs/SetEnabled.h>
30 #include <ff_hw_msgs/SetFlashlight.h>
31 #include <ff_msgs/AckCompletedStatus.h>
32 #include <ff_msgs/AckStamped.h>
33 #include <ff_msgs/AckStatus.h>
34 #include <ff_msgs/AgentStateStamped.h>
35 #include <ff_msgs/ArmAction.h>
36 #include <ff_msgs/CameraStatesStamped.h>
37 #include <ff_msgs/CommandConstants.h>
38 #include <ff_msgs/CommandStamped.h>
39 #include <ff_msgs/CompressedFile.h>
40 #include <ff_msgs/CompressedFileAck.h>
41 #include <ff_msgs/ConfigureCamera.h>
42 #include <ff_msgs/ControlCommand.h>
43 #include <ff_msgs/DockAction.h>
44 #include <ff_msgs/EnableCamera.h>
45 #include <ff_msgs/EnableRecording.h>
46 #include <ff_msgs/FaultState.h>
47 #include <ff_msgs/GuestScienceApk.h>
48 #include <ff_msgs/GuestScienceConfig.h>
49 #include <ff_msgs/GuestScienceState.h>
50 #include <ff_msgs/LocalizationAction.h>
51 #include <ff_msgs/MotionAction.h>
52 #include <ff_msgs/PerchAction.h>
53 #include <ff_msgs/PlanStatusStamped.h>
54 #include <ff_msgs/ResetMap.h>
55 #include <ff_msgs/ResponseOnly.h>
56 #include <ff_msgs/SetDataToDisk.h>
57 #include <ff_msgs/SetExposure.h>
58 #include <ff_msgs/SetFloat.h>
59 #include <ff_msgs/SetInertia.h>
60 #include <ff_msgs/SetRate.h>
61 #include <ff_msgs/SetZones.h>
62 #include <ff_msgs/UnloadLoadNodelet.h>
63 #include <ff_msgs/Zone.h>
71 #include <pluginlib/class_list_macros.h>
73 #include <ros/console.h>
74 #include <ros/package.h>
75 #include <std_srvs/Empty.h>
77 #include <json/json.h>
78 #include <json/value.h>
85 using ff_msgs::CommandConstants;
107 void CmdCallback(ff_msgs::CommandStampedPtr
const& cmd);
119 void InertiaCallback(geometry_msgs::InertiaStampedConstPtr
const& inertia);
123 void PlanCallback(ff_msgs::CompressedFileConstPtr
const& plan);
127 void ZonesCallback(ff_msgs::CompressedFileConstPtr
const& zones);
132 bool FillArmGoal(ff_msgs::CommandStampedPtr
const& cmd);
133 bool FillDockGoal(ff_msgs::CommandStampedPtr
const& cmd,
bool return_to_dock);
135 ff_msgs::CommandStampedPtr
const& cmd =
nullptr);
142 ff_msgs::ArmResultConstPtr
const& result);
145 ff_msgs::DockResultConstPtr
const& result);
149 ff_msgs::LocalizationResultConstPtr
const& result);
153 ff_msgs::MotionResultConstPtr
const& result);
156 ff_msgs::PerchResultConstPtr
const& result);
160 uint8_t completed_status = ff_msgs::AckCompletedStatus::OK,
161 std::string
const& message =
"",
162 uint8_t status = ff_msgs::AckStatus::COMPLETED);
180 std::string
const& current_mobility_state,
181 std::string
const& accepted_mobility_state =
"");
182 bool ArmControl(ff_msgs::CommandStampedPtr
const& cmd);
184 std::string
const& serviceName,
185 std::string
const& cmd_in);
187 std::string
const& cmd_name);
188 bool ConfigureLed(ff_hw_msgs::ConfigureSystemLeds& led_srv);
193 bool PowerItem(ff_msgs::CommandStampedPtr
const& cmd,
bool on);
195 bool ResetEkf(std::string
const& cmd_id);
208 bool AutoReturn(ff_msgs::CommandStampedPtr
const& cmd);
210 bool DeployArm(ff_msgs::CommandStampedPtr
const& cmd);
211 bool Dock(ff_msgs::CommandStampedPtr
const& cmd);
213 bool Fault(ff_msgs::CommandStampedPtr
const& cmd);
217 bool LoadNodelet(ff_msgs::CommandStampedPtr
const& cmd);
218 bool NoOp(ff_msgs::CommandStampedPtr
const& cmd);
219 bool PausePlan(ff_msgs::CommandStampedPtr
const& cmd);
220 bool Perch(ff_msgs::CommandStampedPtr
const& cmd);
221 bool PowerItemOff(ff_msgs::CommandStampedPtr
const& cmd);
222 bool PowerItemOn(ff_msgs::CommandStampedPtr
const& cmd);
223 bool Prepare(ff_msgs::CommandStampedPtr
const& cmd);
225 bool ResetEkf(ff_msgs::CommandStampedPtr
const& cmd);
227 bool RunPlan(ff_msgs::CommandStampedPtr
const& cmd);
228 bool SetCamera(ff_msgs::CommandStampedPtr
const& cmd);
237 bool SetExposure(ff_msgs::CommandStampedPtr
const& cmd);
240 bool SetInertia(ff_msgs::CommandStampedPtr
const& cmd);
241 bool SetMap(ff_msgs::CommandStampedPtr
const& cmd);
243 bool SetPlan(ff_msgs::CommandStampedPtr
const& cmd);
244 bool SetPlanner(ff_msgs::CommandStampedPtr
const& cmd);
246 bool SetZones(ff_msgs::CommandStampedPtr
const& cmd);
247 bool SkipPlanStep(ff_msgs::CommandStampedPtr
const& cmd);
251 bool StopArm(ff_msgs::CommandStampedPtr
const& cmd);
254 bool StowArm(ff_msgs::CommandStampedPtr
const& cmd);
256 bool Undock(ff_msgs::CommandStampedPtr
const& cmd);
258 bool Unperch(ff_msgs::CommandStampedPtr
const& cmd);
259 bool Unterminate(ff_msgs::CommandStampedPtr
const& cmd);
260 bool Wait(ff_msgs::CommandStampedPtr
const& cmd);
267 ff_msgs::CommandStampedPtr cmd);
366 #endif // EXECUTIVE_EXECUTIVE_H_
bool SetZones(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3017
bool StopGuestScience(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3426
ros::Timer sys_monitor_heartbeat_timer_
Definition: executive.h:334
ros::ServiceClient zones_client_
Definition: executive.h:311
bool SetCameraStreaming(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2249
void GuestScienceAckCallback(ff_msgs::AckStampedConstPtr const &ack)
Definition: executive.cc:179
std::string GetRunPlanCmdId()
Definition: executive.cc:1025
Executive()
Definition: executive.cc:27
void DockStateCallback(ff_msgs::DockStateConstPtr const &state)
Definition: executive.cc:127
bool Prepare(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1928
ff_msgs::AckStamped ack_
Definition: executive.h:281
bool ProcessGuestScienceCommand(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1477
ExecutiveActionClient< ff_msgs::PerchAction > perch_ac_
Definition: executive.h:275
bool AutoReturn(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1632
geometry_msgs::InertiaStampedConstPtr current_inertia_
Definition: executive.h:304
ros::Subscriber gs_state_sub_
Definition: executive.h:330
bool Undock(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3513
bool live_led_on_
Definition: executive.h:358
std::shared_ptr< ff_util::ConfigClient > choreographer_cfg_
Definition: executive.h:339
uint8_t GetPlanExecState()
Definition: executive.cc:1021
void GuestScienceStartStopRestartCmdTimeoutCallback(ros::TimerEvent const &te)
Definition: executive.cc:264
bool SetPlanner(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2918
ros::ServiceClient perch_cam_enable_client_
Definition: executive.h:316
bool SetCheckZones(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2369
void PerchResultCallback(ff_util::FreeFlyerActionState::Enum const &state, ff_msgs::PerchResultConstPtr const &result)
Definition: executive.cc:956
~Executive()
Definition: executive.cc:57
void DockResultCallback(ff_util::FreeFlyerActionState::Enum const &state, ff_msgs::DockResultConstPtr const &result)
Definition: executive.cc:865
bool SetCheckObstacles(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2348
bool UnloadNodelet(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3549
ros::Subscriber data_sub_
Definition: executive.h:329
ros::Publisher gs_cmd_pub_
Definition: executive.h:309
ff_msgs::CommandStampedPtr sys_monitor_init_fault_response_
Definition: executive.h:283
bool IsActionRunning(Action action)
Definition: executive.cc:725
sequencer::Sequencer sequencer_
Definition: executive.h:337
bool NoOp(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1875
bool allow_blind_flying_
Definition: executive.h:357
void SetPlanExecState(uint8_t state)
Definition: executive.cc:1074
ff_msgs::DockStateConstPtr dock_state_
Definition: executive.h:290
double motion_feedback_timeout_
Definition: executive.h:349
bool StopArm(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3408
ros::Timer gs_custom_command_timer_
Definition: executive.h:333
ros::Subscriber plan_sub_
Definition: executive.h:329
std::vector< Action > running_actions_
Definition: executive.h:345
bool sys_monitor_heartbeat_fault_blocking_
Definition: executive.h:359
bool FillMotionGoal(Action action, ff_msgs::CommandStampedPtr const &cmd=nullptr)
Definition: executive.cc:645
ros::ServiceClient set_dock_cam_exposure_client_
Definition: executive.h:319
double localization_result_timeout_
Definition: executive.h:351
ros::ServiceClient payload_power_client_
Definition: executive.h:318
bool AreActionsRunning()
Definition: executive.cc:479
std::string gs_custom_cmd_id_
Definition: executive.h:343
Definition: sequencer.h:49
bool StopAllMotion(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3244
OpState * state_
Definition: executive.h:269
ff_msgs::DockGoal dock_goal_
Definition: executive.h:297
ros::Subscriber camera_state_sub_
Definition: executive.h:330
ros::ServiceClient front_flashlight_client_
Definition: executive.h:312
ff_msgs::MotionGoal motion_goal_
Definition: executive.h:299
bool sys_monitor_init_fault_blocking_
Definition: executive.h:360
bool SetEnableAutoReturn(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2520
double dock_result_timeout_
Definition: executive.h:350
bool sys_monitor_init_fault_occurring_
Definition: executive.h:362
double sys_monitor_startup_time_secs_
Definition: executive.h:352
ros::ServiceClient dock_cam_enable_client_
Definition: executive.h:313
ros::ServiceClient perch_cam_config_client_
Definition: executive.h:316
void LedConnectedCallback()
Definition: executive.cc:282
ros::Subscriber perch_state_sub_
Definition: executive.h:331
double led_connected_timeout_
Definition: executive.h:351
ff_msgs::MobilityState GetMobilityState()
Definition: executive.cc:1017
void SetMobilityState()
Definition: executive.cc:1031
ros::ServiceClient dock_cam_config_client_
Definition: executive.h:313
Action
Definition: executive_action_client.h:29
ff_msgs::AgentStateStamped agent_state_
Definition: executive.h:279
bool SkipPlanStep(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3170
ros::ServiceClient laser_enable_client_
Definition: executive.h:311
bool PausePlan(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1881
std::shared_ptr< ff_util::ConfigClient > mapper_cfg_
Definition: executive.h:340
ff_msgs::CommandStampedPtr GetPlanCommand()
Definition: executive.cc:1582
ros::Subscriber gs_ack_sub_
Definition: executive.h:328
bool RemoveAction(Action action)
Definition: executive.cc:818
std::string primary_apk_running_
Definition: executive.h:342
void StartWaitTimer(float duration)
Definition: executive.cc:1561
ff_msgs::LocalizationGoal localization_goal_
Definition: executive.h:298
ros::Subscriber cmd_sub_
Definition: executive.h:328
bool ResetEkf(std::string const &cmd_id)
Definition: executive.cc:1553
bool CheckServiceExists(ros::ServiceClient &serviceIn, std::string const &serviceName, std::string const &cmd_in)
Definition: executive.cc:1135
void SetOpState(OpState *state)
Definition: executive.cc:1063
ff_msgs::CompressedFileConstPtr zones_
Definition: executive.h:287
void MotionStateCallback(ff_msgs::MotionStatePtr const &state)
Definition: executive.cc:300
void PublishCmdAck(std::string const &cmd_id, uint8_t completed_status=ff_msgs::AckCompletedStatus::OK, std::string const &message="", uint8_t status=ff_msgs::AckStatus::COMPLETED)
Definition: executive.cc:983
bool FillArmGoal(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:536
void FaultStateCallback(ff_msgs::FaultStateConstPtr const &state)
Definition: executive.cc:145
void WaitCallback(ros::TimerEvent const &te)
Definition: executive.cc:465
ros::ServiceClient nav_cam_config_client_
Definition: executive.h:315
bool FailCommandIfMoving(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1245
bool ConfigureMobility(bool move_to_start, std::string &err_msg)
Definition: executive.cc:1178
bool Wait(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3617
bool ReacquirePosition(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1941
void AckMobilityStateIssue(ff_msgs::CommandStampedPtr const &cmd, std::string const ¤t_mobility_state, std::string const &accepted_mobility_state="")
Definition: executive.cc:1086
bool Unterminate(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3589
int pub_queue_size_
Definition: executive.h:354
ros::Subscriber motion_sub_
Definition: executive.h:329
void DataToDiskCallback(ff_msgs::CompressedFileConstPtr const &data)
Definition: executive.cc:118
double sys_monitor_heartbeat_timeout_
Definition: executive.h:352
void PublishPlan()
Definition: executive.cc:1002
void MotionFeedbackCallback(ff_msgs::MotionFeedbackConstPtr const &feedback)
Definition: executive.cc:921
double gs_command_timeout_
Definition: executive.h:348
bool SetCameraRecording(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2150
ros::Time MsToSec(std::string timestamp)
Definition: executive.cc:1366
ros::Subscriber dock_state_sub_
Definition: executive.h:328
bool RunPlan(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1968
ExecutiveActionClient< ff_msgs::MotionAction > motion_ac_
Definition: executive.h:274
bool ReadCommand(config_reader::ConfigReader::Table *response, ff_msgs::CommandStampedPtr cmd)
Definition: executive.cc:4207
void ArmResultCallback(ff_util::FreeFlyerActionState::Enum const &state, ff_msgs::ArmResultConstPtr const &result)
Definition: executive.cc:839
std::string run_plan_cmd_id_
Definition: executive.h:342
bool GripperControl(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1799
bool SetMap(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2793
bool SetTelemetryRate(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2950
ros::ServiceClient reset_map_client_
Definition: executive.h:323
bool SetHolonomicMode(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2714
ff_msgs::CompressedFileAck cf_ack_
Definition: executive.h:286
bool SetEnableReplan(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2557
ros::ServiceClient sci_cam_config_client_
Definition: executive.h:317
ros::ServiceClient enable_recording_client_
Definition: executive.h:322
bool Unperch(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3554
ros::Publisher cmd_ack_pub_
Definition: executive.h:308
bool PowerItem(ff_msgs::CommandStampedPtr const &cmd, bool on)
Definition: executive.cc:1375
ros::Timer reload_params_timer_
Definition: executive.h:334
void SetRunPlanCmdId(std::string cmd_id)
Definition: executive.cc:1079
ros::ServiceClient nav_cam_enable_client_
Definition: executive.h:315
ros::ServiceClient enable_astrobee_intercommunication_client_
Definition: executive.h:324
ros::Publisher plan_pub_
Definition: executive.h:308
ItemType
Definition: sequencer.h:43
bool ArmPanAndTilt(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1627
Definition: ff_nodelet.h:57
bool Perch(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1886
ros::Subscriber inertia_sub_
Definition: executive.h:331
bool GetSetPlanInertia(std::string const &cmd_id)
Definition: executive.cc:1586
void PlanCallback(ff_msgs::CompressedFileConstPtr const &plan)
Definition: executive.cc:379
bool SwitchLocalization(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3475
bool StartAction(Action action, std::string const &cmd_id)
Definition: executive.cc:734
ros::ServiceClient eps_terminate_client_
Definition: executive.h:323
bool ArmControl(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1100
bool StopRecording(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3431
void PublishPlanStatus(uint8_t status)
Definition: executive.cc:1006
ff_msgs::CompressedFileConstPtr data_to_disk_
Definition: executive.h:287
Definition: config_reader.h:48
ff_msgs::GuestScienceConfigConstPtr guest_science_config_
Definition: executive.h:292
ff_msgs::MotionStatePtr motion_state_
Definition: executive.h:293
ff_msgs::ArmGoal arm_goal_
Definition: executive.h:296
bool StowArm(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3462
bool StartRecording(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3199
ros::Publisher agent_state_pub_
Definition: executive.h:308
bool IdlePropulsion(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1804
std::unique_ptr< Executive > ExecutivePtr
Definition: executive.h:364
void StopWaitTimer()
Definition: executive.cc:1566
ros::ServiceClient set_rate_client_
Definition: executive.h:321
void GuestScienceStateCallback(ff_msgs::GuestScienceStateConstPtr const &state)
Definition: executive.cc:202
void SysMonitorHeartbeatCallback(ff_msgs::HeartbeatConstPtr const &heartbeat)
Definition: executive.cc:387
bool SetInertia(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2734
bool SetCamera(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1994
bool SetPlan(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2877
ff_msgs::CompressedFileConstPtr plan_
Definition: executive.h:287
ros::Timer gs_start_stop_restart_command_timer_
Definition: executive.h:333
bool SetDataToDisk(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2390
bool SetExposure(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2577
Enum
Definition: ff_action.h:41
ros::ServiceClient set_inertia_client_
Definition: executive.h:321
bool RestartGuestScience(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1963
ff_msgs::PerchStateConstPtr perch_state_
Definition: executive.h:294
ros::ServiceClient pmc_enable_client_
Definition: executive.h:318
ff_msgs::CameraStatesStamped camera_states_
Definition: executive.h:289
ros::NodeHandle nh_
Definition: executive.h:306
bool LoadUnloadNodelet(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1276
ExecutiveActionClient< ff_msgs::ArmAction > arm_ac_
Definition: executive.h:271
bool SetEnableImmediate(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2537
ros::Publisher cf_ack_pub_
Definition: executive.h:309
double arm_feedback_timeout_
Definition: executive.h:349
ff_msgs::FaultStateConstPtr fault_state_
Definition: executive.h:291
ros::ServiceClient set_collision_distance_client_
Definition: executive.h:326
std::string gs_start_stop_restart_cmd_id_
Definition: executive.h:343
bool AckCurrentPlanItem()
Definition: executive.cc:1572
bool SetOperatingLimits(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2826
ros::Subscriber gs_config_sub_
Definition: executive.h:330
ros::ServiceClient sci_cam_enable_client_
Definition: executive.h:317
Definition: config_reader.h:54
ros::ServiceClient unload_load_nodelet_client_
Definition: executive.h:325
virtual void Initialize(ros::NodeHandle *nh)
Definition: executive.cc:3632
void PublishAgentState()
Definition: executive.cc:4342
bool DeployArm(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1698
bool sys_monitor_heartbeat_fault_occurring_
Definition: executive.h:361
void LocalizationResultCallback(ff_util::FreeFlyerActionState::Enum const &state, ff_msgs::LocalizationResultConstPtr const &result)
Definition: executive.cc:891
bool ConfigureLed(ff_hw_msgs::ConfigureSystemLeds &led_srv)
Definition: executive.cc:1161
void MotionResultCallback(ff_util::FreeFlyerActionState::Enum const &state, ff_msgs::MotionResultConstPtr const &result)
Definition: executive.cc:930
bool SetFlashlightBrightness(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:2640
ExecutiveActionClient< ff_msgs::LocalizationAction > localization_ac_
Definition: executive.h:273
void CameraStatesCallback(ff_msgs::CameraStatesStampedConstPtr const &state)
Definition: executive.cc:61
ros::Timer wait_timer_
Definition: executive.h:334
void CancelAction(Action action, std::string cmd)
Definition: executive.cc:486
Definition: executive.h:100
bool ReadParams()
Definition: executive.cc:4005
ros::Subscriber heartbeat_sub_
Definition: executive.h:329
uint8_t state
Definition: signal_lights.h:90
ros::Subscriber fault_state_sub_
Definition: executive.h:328
bool Dock(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1711
double action_active_timeout_
Definition: executive.h:348
ros::ServiceClient haz_cam_config_client_
Definition: executive.h:314
ros::Subscriber zones_sub_
Definition: executive.h:329
ros::ServiceClient haz_cam_enable_client_
Definition: executive.h:314
void GuestScienceConfigCallback(ff_msgs::GuestScienceConfigConstPtr const &config)
Definition: executive.cc:197
bool CustomGuestScience(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1671
bool Fault(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1777
Definition: executive.h:87
ff_msgs::CommandStampedPtr sys_monitor_heartbeat_fault_response_
Definition: executive.h:284
void ZonesCallback(ff_msgs::CompressedFileConstPtr const &zones)
Definition: executive.cc:470
config_reader::ConfigReader mapper_config_params_
Definition: executive.h:277
void CmdCallback(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:98
bool LoadNodelet(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1870
ros::Publisher plan_status_pub_
Definition: executive.h:308
ExecutiveActionClient< ff_msgs::DockAction > dock_ac_
Definition: executive.h:272
bool EnableAstrobeeIntercomms(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1745
double Time
Definition: time.h:23
void PerchStateCallback(ff_msgs::PerchStateConstPtr const &state)
Definition: executive.cc:350
bool FillDockGoal(ff_msgs::CommandStampedPtr const &cmd, bool return_to_dock)
Definition: executive.cc:602
void SysMonitorTimeoutCallback(ros::TimerEvent const &te)
Definition: executive.cc:450
ros::Timer sys_monitor_startup_timer_
Definition: executive.h:335
bool PowerItemOn(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1923
int sub_queue_size_
Definition: executive.h:355
ros::ServiceClient set_nav_cam_exposure_client_
Definition: executive.h:320
bool PowerItemOff(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1918
sequencer::ItemType GetCurrentPlanItemType()
Definition: executive.cc:1578
void GuestScienceCustomCmdTimeoutCallback(ros::TimerEvent const &te)
Definition: executive.cc:252
ros::ServiceClient set_data_client_
Definition: executive.h:322
void GetSetPlanOperatingLimits()
Definition: executive.cc:1616
ros::ServiceClient back_flashlight_client_
Definition: executive.h:312
double perch_result_timeout_
Definition: executive.h:350
bool ReadMapperParams()
Definition: executive.cc:4147
Definition: op_state.h:45
void InertiaCallback(geometry_msgs::InertiaStampedConstPtr const &inertia)
Definition: executive.cc:277
ff_msgs::PerchGoal perch_goal_
Definition: executive.h:300
bool StartGuestScience(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:3194
config_reader::ConfigReader config_params_
Definition: executive.h:277
ff_util::FreeFlyerServiceClient< ff_hw_msgs::ConfigureSystemLeds > led_client_
Definition: executive.h:302
bool InitializeBias(ff_msgs::CommandStampedPtr const &cmd)
Definition: executive.cc:1850
bool CheckStoppedOrDrifting(std::string const &cmd_id, std::string const &cmd_name)
Definition: executive.cc:1147