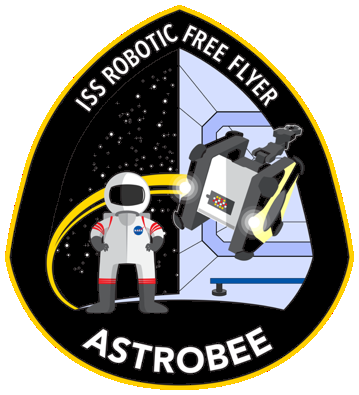 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef CTL_CTL_ROS_H_
20 #define CTL_CTL_ROS_H_
28 #include <geometry_msgs/InertiaStamped.h>
29 #include <geometry_msgs/PoseStamped.h>
30 #include <geometry_msgs/TwistStamped.h>
33 #include <std_srvs/SetBool.h>
36 #include <ff_msgs/ControlAction.h>
39 #include <ff_msgs/ControlCommand.h>
40 #include <ff_msgs/FlightMode.h>
41 #include <ff_msgs/EkfState.h>
89 explicit Ctl(ros::NodeHandle* nh, std::string
const&
name);
101 bool EnableCtl(std_srvs::SetBoolRequest&req, std_srvs::SetBoolResponse &response);
112 void PoseCallback(
const geometry_msgs::PoseStamped::ConstPtr& truth);
115 void TwistCallback(
const geometry_msgs::TwistStamped::ConstPtr& truth);
118 void InertiaCallback(
const geometry_msgs::InertiaStamped::ConstPtr& inertia);
135 void GoalCallback(ff_msgs::ControlGoalConstPtr
const& control_goal);
147 ff_msgs::ControlCommand
const& poseVel = ff_msgs::ControlCommand());
162 std::mutex mutex_cmd_msg_, mutex_segment_;
164 ros::Subscriber truth_pose_sub_, inertia_sub_, flight_mode_sub_;
165 ros::Subscriber twist_sub_, pose_sub_, ekf_sub_, command_sub_;
166 ros::Publisher ctl_pub_, traj_pub_, segment_pub_, progress_pub_;
167 ros::ServiceServer enable_srv_;
173 ff_util::Segment::iterator setpoint_;
174 ff_msgs::ControlFeedback feedback_;
175 ff_msgs::ControlCommand command_;
179 ros::Timer config_timer_;
185 bool inertia_received_;
186 bool control_enabled_;
187 bool flight_enabled_;
189 float stopping_vel_thresh_squared_;
190 float stopping_omega_thresh_squared_;
197 #endif // CTL_CTL_ROS_H_
uint8_t mode
Definition: signal_lights.h:74
Ctl(ros::NodeHandle *nh, std::string const &name)
Definition: ctl_ros.cc:39
void PoseCallback(const geometry_msgs::PoseStamped::ConstPtr &truth)
Definition: ctl_ros.cc:270
@ NOMINAL
Definition: ctl_ros.h:71
void GoalCallback(ff_msgs::ControlGoalConstPtr const &control_goal)
Definition: ctl_ros.cc:386
void CancelCallback()
Definition: ctl_ros.cc:434
void SetpointCallback(const ff_msgs::ControlState::ConstPtr &command)
Definition: ctl_ros.cc:318
~Ctl()
Definition: ctl_ros.cc:153
void EkfCallback(const ff_msgs::EkfState::ConstPtr &state)
Definition: ctl_ros.cc:235
@ STOPPING
Definition: ctl_ros.h:72
@ WAITING
Definition: ctl_ros.h:70
std::string getName()
Definition: ctl_ros.cc:560
@ GOAL_CANCEL
Definition: ctl_ros.h:79
Definition: perf_timer.h:31
void ControlTimerCallback(const ros::TimerEvent &event)
@ GOAL_COMPLETE
Definition: ctl_ros.h:77
std::vector< Setpoint > Segment
Definition: ff_flight.h:47
void TwistCallback(const geometry_msgs::TwistStamped::ConstPtr &truth)
Definition: ctl_ros.cc:285
void PreemptCallback()
Definition: ctl_ros.cc:440
uint32_t Event
Definition: ff_fsm.h:33
void ReadParams(void)
Definition: ctl_ros.cc:563
std::string name
Definition: eps_simulator.cc:48
@ GOAL_STOP
Definition: ctl_ros.h:80
void UpdateCallback(FSM::State const &state, FSM::Event const &event)
Definition: ctl_ros.cc:215
void TimerCallback(const ros::TimerEvent &event)
Definition: ctl_ros.cc:335
bool EnableCtl(std_srvs::SetBoolRequest &req, std_srvs::SetBoolResponse &response)
Definition: ctl_ros.cc:192
Definition: config_reader.h:48
int8_t State
Definition: ff_fsm.h:32
bool Step(ros::Time curr_time)
Definition: ctl_ros.cc:472
void InertiaCallback(const geometry_msgs::InertiaStamped::ConstPtr &inertia)
Definition: ctl_ros.cc:296
FSM::State Result(int32_t response)
Definition: ctl_ros.cc:155
void FlightModeCallback(const ff_msgs::FlightMode::ConstPtr &mode)
Definition: ctl_ros.cc:305
uint8_t state
Definition: signal_lights.h:90
ff_util::FSM FSM
Definition: ctl_ros.h:60
@ GOAL_NOMINAL
Definition: ctl_ros.h:78
double Time
Definition: time.h:23
Controller implementation using GNC module.
Definition: ctl_ros.h:66
bool Command(uint8_t const mode, ff_msgs::ControlCommand const &poseVel=ff_msgs::ControlCommand())
Definition: ctl_ros.cc:446
static constexpr double MAX_LATENCY
Definition: ctl_ros.h:84