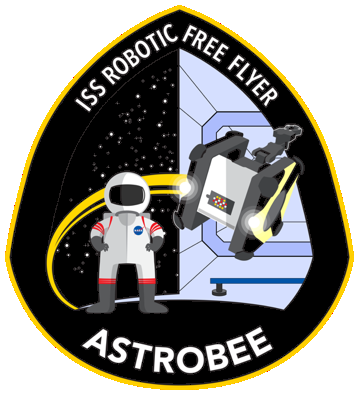 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
40 #ifndef POINT_CLOUD_COMMON_ORGANIZED_NEIGHBOR2_H_
41 #define POINT_CLOUD_COMMON_ORGANIZED_NEIGHBOR2_H_
43 #include <pcl/point_cloud.h>
44 #include <pcl/point_types.h>
45 #include <pcl/search/search.h>
46 #include <pcl/common/eigen.h>
59 template <
typename Po
intT>
69 typedef boost::shared_ptr<pcl::search::OrganizedNeighbor2<PointT> >
Ptr;
70 typedef boost::shared_ptr<const pcl::search::OrganizedNeighbor2<PointT> >
ConstPtr;
72 using pcl::search::Search<PointT>::indices_;
73 using pcl::search::Search<PointT>::sorted_results_;
74 using pcl::search::Search<PointT>::input_;
81 : Search<PointT>(
"OrganizedNeighbor2", sorted_results),
mask_() {}
93 mask_.resize(input_->size());
97 if (indices_.get() != NULL && indices_->size() != 0) {
98 mask_.assign(input_->size(), 0);
99 for (std::vector<int>::const_iterator iIt = indices_->begin(); iIt != indices_->end(); ++iIt)
mask_[*iIt] = 1;
101 mask_.assign(input_->size(), 1);
115 int radiusSearch(
const PointT& p_q,
double radius, std::vector<int>& k_indices, std::vector<float>& k_sqr_distances,
116 unsigned int max_nn = 0)
const;
126 int nearestKSearch(
const PointT& p_q,
int k, std::vector<int>& k_indices, std::vector<float>& k_sqr_distances)
const;
132 Eigen::Vector2f
projectPoint(
const Eigen::Vector3f& p)
const;
156 inline bool testPoint(
const PointT& query,
unsigned k, std::priority_queue<Entry>& queue,
unsigned index)
const {
157 const PointT& point = input_->points[index];
158 if (
mask_[index] && pcl_isfinite(point.x)) {
160 float dist_x = point.x - query.x;
161 float dist_y = point.y - query.y;
162 float dist_z = point.z - query.z;
163 float squared_distance = dist_x * dist_x + dist_y * dist_y + dist_z * dist_z;
164 if (queue.size() < k) {
165 queue.push(
Entry(index, squared_distance));
166 }
else if (queue.top().distance > squared_distance) {
168 queue.push(
Entry(index, squared_distance));
175 inline void clipRange(
int& begin,
int& end,
int min,
int max)
const {
176 begin = std::max(std::min(begin, max), min);
177 end = std::min(std::max(end, min), max);
189 unsigned& maxX,
unsigned& maxY)
const;
200 EIGEN_MAKE_ALIGNED_OPERATOR_NEW
205 #ifdef PCL_NO_PRECOMPILE
209 #endif // POINT_CLOUD_COMMON_ORGANIZED_NEIGHBOR2_H_
boost::shared_ptr< const pcl::search::OrganizedNeighbor2< PointT > > ConstPtr
Definition: organized_neighbor2.h:70
Definition: correspondence_rejection_surface_normal2.h:45
unsigned index
Definition: organized_neighbor2.h:143
virtual ~OrganizedNeighbor2()
Empty deconstructor.
Definition: organized_neighbor2.h:84
pcl::PointCloud< PointT > PointCloud
Definition: organized_neighbor2.h:63
Eigen::Matrix3f KR_KRT_
Definition: organized_neighbor2.h:194
Eigen::Matrix3f intrinsics_matrix_
the intrinsics matrix.
Definition: organized_neighbor2.h:192
void clipRange(int &begin, int &end, int min, int max) const
Definition: organized_neighbor2.h:175
Entry()
Definition: organized_neighbor2.h:142
boost::shared_ptr< PointCloud > PointCloudPtr
Definition: organized_neighbor2.h:64
bool operator<(const Entry &other) const
Definition: organized_neighbor2.h:146
boost::shared_ptr< const std::vector< int > > IndicesConstPtr
Definition: organized_neighbor2.h:67
Entry(int idx, float dist)
Definition: organized_neighbor2.h:141
void getProjectedRadiusSearchBox(const PointT &point, float squared_radius, unsigned &minX, unsigned &minY, unsigned &maxX, unsigned &maxY) const
Obtain a search box in 2D from a sphere with a radius in 3D.
Definition: organized_neighbor2_impl.h:242
bool testPoint(const PointT &query, unsigned k, std::priority_queue< Entry > &queue, unsigned index) const
test if point given by index is among the k NN in results to the query point.
Definition: organized_neighbor2.h:156
void setIntrinsicsMatrix(const Eigen::Matrix3d &intrinsics_matrix)
Definition: organized_neighbor2.h:134
std::vector< unsigned char > mask_
mask, indicating whether the point was in the indices list or not.
Definition: organized_neighbor2.h:197
virtual void setInputCloud(const PointCloudConstPtr &cloud, const IndicesConstPtr &indices=IndicesConstPtr())
Provide a pointer to the input data set, if user has focal length he must set it before calling this.
Definition: organized_neighbor2.h:90
boost::shared_ptr< const PointCloud > PointCloudConstPtr
Definition: organized_neighbor2.h:66
OrganizedNeighbor2(bool sorted_results=false)
Constructor.
Definition: organized_neighbor2.h:80
int nearestKSearch(const PointT &p_q, int k, std::vector< int > &k_indices, std::vector< float > &k_sqr_distances) const
Search for the k-nearest neighbors for a given query point.
Definition: organized_neighbor2_impl.h:113
Definition: organized_neighbor2.h:140
int radiusSearch(const PointT &p_q, double radius, std::vector< int > &k_indices, std::vector< float > &k_sqr_distances, unsigned int max_nn=0) const
Search for all neighbors of query point that are within a given radius.
Definition: organized_neighbor2_impl.h:55
boost::shared_ptr< pcl::search::OrganizedNeighbor2< PointT > > Ptr
Definition: organized_neighbor2.h:69
OrganizedNeighbor is a class for optimized nearest neigbhor search in organized point clouds.
Definition: organized_neighbor2.h:60
float distance
Definition: organized_neighbor2.h:144
Eigen::Vector2f projectPoint(const Eigen::Vector3f &p) const
projects a point into the image
Definition: organized_neighbor2_impl.h:287