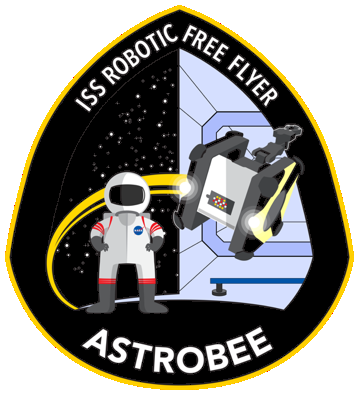 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef JSONLOADER_KEEPOUT_H_
20 #define JSONLOADER_KEEPOUT_H_
22 #include <Eigen/Geometry>
35 explicit Keepout(
bool const safe);
44 bool IsSafe() const noexcept;
53 #endif // JSONLOADER_KEEPOUT_H_
Keepout(Sequence const &boxes, bool const safe)
Definition: keepout.cc:23
bool IsSafe() const noexcept
Definition: keepout.cc:36
Sequence const & GetBoxes() const
Definition: keepout.cc:32
Eigen::AlignedBox3f BoundingBox
Definition: keepout.h:31
std::vector< BoundingBox > Sequence
Definition: keepout.h:32
void Merge(Keepout const &zone)
Definition: keepout.cc:40