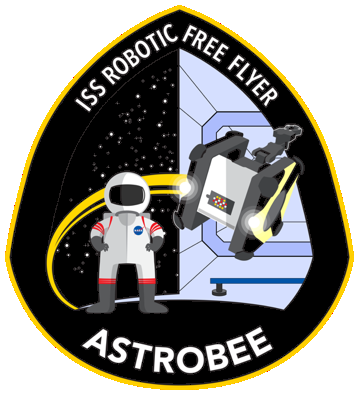 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef GRAPH_FACTORS_INVERSE_DEPTH_PROJECTION_FACTOR_H_
20 #define GRAPH_FACTORS_INVERSE_DEPTH_PROJECTION_FACTOR_H_
24 #include <gtsam/geometry/Pose3.h>
25 #include <gtsam/nonlinear/NonlinearFactor.h>
26 #include <boost/optional.hpp>
42 typedef NoiseModelFactor3<Pose3, vision_common::InverseDepthMeasurement, Pose3>
Base;
43 typedef boost::shared_ptr<InverseDepthProjectionFactor>
shared_ptr;
58 Key inverseDepthMeasurementKey, Key targetPoseKey)
59 :
Base(model, sourcePoseKey, inverseDepthMeasurementKey, targetPoseKey),
76 :
Base(model, sourcePoseKey, inverseDepthCameraPoseKey, targetPoseKey),
83 virtual NonlinearFactor::shared_ptr
clone()
const {
84 return boost::static_pointer_cast<NonlinearFactor>(NonlinearFactor::shared_ptr(
new This(*
this)));
92 void print(
const std::string& s =
"",
const KeyFormatter& keyFormatter = DefaultKeyFormatter)
const override {
93 std::cout << s <<
"InverseDepthProjectionFactor, z = ";
95 Base::print(
"", keyFormatter);
98 bool equals(
const NonlinearFactor& p,
double tol = 1e-9)
const override {
99 const This* e =
dynamic_cast<const This*
>(&p);
100 return e && Base::equals(p, tol) && traits<Point2>::Equals(this->measured_, e->
measured_, tol);
106 const Pose3& world_T_target,
107 boost::optional<Matrix&> d_projected_point_d_world_T_source = boost::none,
108 boost::optional<Matrix&> d_projected_point_d_inverse_depth = boost::none,
109 boost::optional<Matrix&> d_projected_point_d_world_T_target = boost::none)
const override {
110 const auto projected_measurement =
111 inverseDepthMeasurement.
Project(world_T_source, world_T_target, d_projected_point_d_world_T_source,
112 d_projected_point_d_world_T_target, d_projected_point_d_inverse_depth);
113 if (!projected_measurement) {
114 if (d_projected_point_d_world_T_source) *d_projected_point_d_world_T_source = Matrix::Zero(2, 6);
115 if (d_projected_point_d_world_T_target) *d_projected_point_d_world_T_target = Matrix::Zero(2, 6);
116 if (d_projected_point_d_inverse_depth) *d_projected_point_d_inverse_depth = Matrix::Zero(2, 1);
118 std::cout <<
"Landmark moved behind camera " << DefaultKeyFormatter(this->key2()) << std::endl;
119 return Vector2::Constant(0.0);
121 return *projected_measurement -
measured_;
131 template <
class ARCHIVE>
132 void serialize(ARCHIVE& ar,
const unsigned int ) {
133 ar& BOOST_SERIALIZATION_BASE_OBJECT_NVP(
Base);
139 EIGEN_MAKE_ALIGNED_OPERATOR_NEW
148 #endif // GRAPH_FACTORS_INVERSE_DEPTH_PROJECTION_FACTOR_H_
bool equals(const NonlinearFactor &p, double tol=1e-9) const override
Definition: inverse_depth_projection_factor.h:98
NoiseModelFactor3< Pose3, vision_common::InverseDepthMeasurement, Pose3 > Base
Definition: inverse_depth_projection_factor.h:42
boost::optional< Eigen::Vector2d > Project(const gtsam::Pose3 &world_T_source_body, const gtsam::Pose3 &world_T_target_body, boost::optional< gtsam::Matrix & > d_projected_point_d_world_T_source_body=boost::none, boost::optional< gtsam::Matrix & > d_projected_point_d_world_T_target_body=boost::none, boost::optional< gtsam::Matrix & > d_projected_point_d_inverse_depth=boost::none) const
Definition: inverse_depth_measurement.h:58
bool verboseCheirality() const
Definition: inverse_depth_projection_factor.h:126
Point2 measured_
Definition: inverse_depth_projection_factor.h:38
Vector evaluateError(const Pose3 &world_T_source, const vision_common::InverseDepthMeasurement &inverseDepthMeasurement, const Pose3 &world_T_target, boost::optional< Matrix & > d_projected_point_d_world_T_source=boost::none, boost::optional< Matrix & > d_projected_point_d_inverse_depth=boost::none, boost::optional< Matrix & > d_projected_point_d_world_T_target=boost::none) const override
Evaluate error h(x)-z and optionally derivatives.
Definition: inverse_depth_projection_factor.h:104
InverseDepthProjectionFactor(const Point2 &measured, const SharedNoiseModel &model, Key sourcePoseKey, Key inverseDepthMeasurementKey, Key targetPoseKey)
Definition: inverse_depth_projection_factor.h:57
InverseDepthProjectionFactor(const Point2 &measured, const SharedNoiseModel &model, Key sourcePoseKey, Key inverseDepthCameraPoseKey, Key targetPoseKey, bool verboseCheirality)
Definition: inverse_depth_projection_factor.h:74
Definition: inverse_depth_projection_factor.h:36
const Point2 & measured() const
Definition: inverse_depth_projection_factor.h:124
virtual ~InverseDepthProjectionFactor()
Definition: inverse_depth_projection_factor.h:80
friend class boost::serialization::access
Serialization function.
Definition: inverse_depth_projection_factor.h:130
Definition: cumulative_factor.h:26
InverseDepthProjectionFactor This
Definition: inverse_depth_projection_factor.h:44
boost::shared_ptr< InverseDepthProjectionFactor > shared_ptr
Definition: inverse_depth_projection_factor.h:43
bool verboseCheirality_
Definition: inverse_depth_projection_factor.h:39
virtual NonlinearFactor::shared_ptr clone() const
Definition: inverse_depth_projection_factor.h:83
void print(const std::string &s="", const KeyFormatter &keyFormatter=DefaultKeyFormatter) const override
Definition: inverse_depth_projection_factor.h:92
Definition: inverse_depth_measurement.h:40
InverseDepthProjectionFactor()
Definition: inverse_depth_projection_factor.h:46