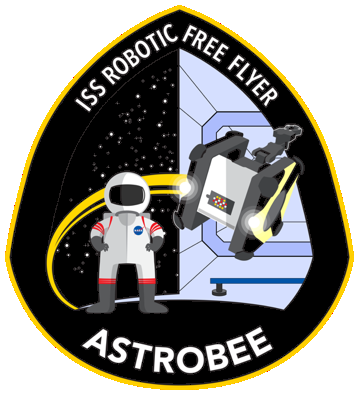 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef I2C_I2C_NEW_H_
20 #define I2C_I2C_NEW_H_
41 using Ptr = std::shared_ptr<Bus>;
44 Bus(
Bus const& other) =
delete;
53 const std::uint8_t* data,
const std::size_t size,
57 std::uint8_t *data,
const std::size_t size,
60 std::uint8_t *data,
const std::size_t size,
68 bool SetAddress(
const Address addr) noexcept;
87 explicit operator bool()
const noexcept;
89 int Write(
const std::uint8_t* data,
const std::size_t size,
93 int WriteRegister(
const std::uint8_t reg,
const std::uint8_t data,
96 const std::uint8_t *data,
const std::size_t size,
99 int Read(std::uint8_t *data,
const std::size_t size,
102 std::uint8_t *data,
const std::size_t size,
116 #endif // I2C_I2C_NEW_H_
int Write(const std::uint8_t *data, const std::size_t size, Error *ec)
Definition: i2c_new.cc:195
void SetRetries(const int retries) noexcept
Definition: i2c_new.cc:171
Bus(Bus const &other)=delete
std::uint16_t Address
Definition: i2c_new.h:31
int Read(const Address addr, std::uint8_t *data, const std::size_t size, Error *ec)
Definition: i2c_new.cc:115
std::shared_ptr< Bus > Ptr
Definition: i2c_new.h:41
Device DeviceAt(const Address addr) const noexcept
Definition: i2c_new.cc:78
int Read(std::uint8_t *data, const std::size_t size, Error *ec)
Definition: i2c_new.cc:241
friend Bus::Ptr Open(std::string const &, Error *)
Bus::Ptr Open(std::string const &dev, Error *err)
Definition: i2c_new.cc:46
int WriteRegister(const std::uint8_t reg, const std::uint8_t data, Error *ec)
Definition: i2c_new.cc:204
int ReadRegister(const std::uint8_t reg, std::uint8_t *data, const std::size_t size, Error *ec)
Definition: i2c_new.cc:245
int Write(const Address addr, const std::uint8_t *data, const std::size_t size, Error *ec)
Definition: i2c_new.cc:92
i2c::Address addr() const noexcept
Definition: i2c_new.cc:187
Bus & operator=(Bus const &other)=delete
int Error
Definition: i2c_new.h:30
int ReadRegister(const Address addr, const std::uint8_t reg, std::uint8_t *data, const std::size_t size, Error *ec)
Definition: i2c_new.cc:138
const Bus::Ptr & bus() const noexcept
Definition: i2c_new.cc:191
~Bus()
Definition: i2c_new.cc:87
std::weak_ptr< Bus > WeakPtr
Definition: i2c_new.h:42