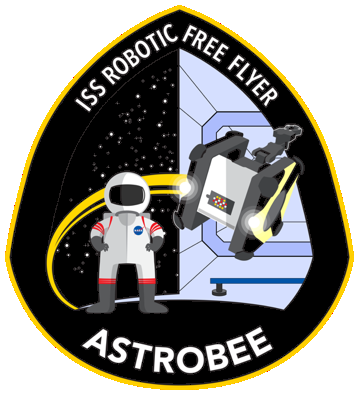 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_PRO_GUROBI_SOLVER_H_
20 #define TRAJ_OPT_PRO_GUROBI_SOLVER_H_
34 const std::vector<Waypoint> &waypnts,
const std::vector<MatD> &A,
35 const std::vector<VecD> &b,
const std::vector<decimal_t> &ds,
37 boost::shared_ptr<Vec3Vec> points = boost::shared_ptr<Vec3Vec>(),
40 void addPathCost(
const std::vector<MatD> &A,
const std::vector<VecD> &b,
44 const std::vector<decimal_t> &ds);
45 void seedDs(boost::shared_ptr<Vec3Vec> points, std::vector<decimal_t> *dsp);
46 void sikangSeed(boost::shared_ptr<Vec3Vec> points,
47 std::vector<decimal_t> *dsp,
decimal_t v0,
60 boost::shared_ptr<LegendreTrajectory> ltraj_;
63 boost::shared_ptr<GRBEnv> grb_env_;
64 bool initializeGurobi();
66 void addLineCost(boost::shared_ptr<Vec3Vec> points,
double upsilon);
73 #endif // TRAJ_OPT_PRO_GUROBI_SOLVER_H_
uint8_t mode
Definition: signal_lights.h:74
PolyType
Definition: traj_data.h:27
Eigen::Matrix< decimal_t, Eigen::Dynamic, Eigen::Dynamic > MatD
Definition: types.h:51
void seedDs(boost::shared_ptr< Vec3Vec > points, std::vector< decimal_t > *dsp)
void sikangSeed(boost::shared_ptr< Vec3Vec > points, std::vector< decimal_t > *dsp, decimal_t v0, decimal_t ratio=1.0)
decimal_t getCost()
Definition: gurobi_solver.h:55
void addPathCost(const std::vector< MatD > &A, const std::vector< VecD > &b, double epsilon)
ConstraintMode
Definition: gurobi_trajectory.h:119
double decimal_t
Definition: types.h:35
bool checkMax(decimal_t r)
Definition: msg_traj.h:27
void setConParam(ConstraintMode mode, int num)
Definition: gurobi_solver.h:51
Definition: trajectory_solver.h:46
bool solveTrajectory(const std::vector< Waypoint > &waypnts, const std::vector< MatD > &A, const std::vector< VecD > &b, const std::vector< decimal_t > &ds, decimal_t epsilon=0, boost::shared_ptr< Vec3Vec > points=boost::shared_ptr< Vec3Vec >(), decimal_t upsilon=0)
Definition: gurobi_solver.h:30
bool use_lp_
Definition: gurobi_solver.h:49
bool solveConvexifyed(const std::vector< Waypoint > &waypnts, const MatD &Aobs, const VecD &bobs, const MatD &Aenv, const VecD &benv, const std::vector< decimal_t > &ds)
Eigen::Matrix< decimal_t, Eigen::Dynamic, 1 > VecD
Definition: types.h:49
void setPolyParams(int degree, PolyType type, int order)
boost::shared_ptr< Trajectory > getTrajectory()