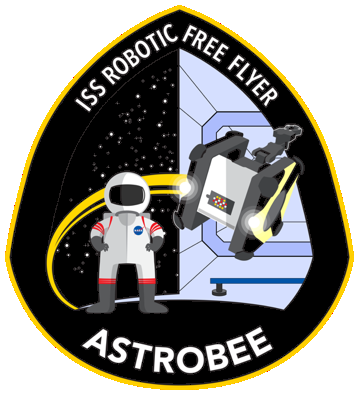 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FLASHLIGHT_FLASHLIGHT_H_
20 #define FLASHLIGHT_FLASHLIGHT_H_
26 enum class Mode : std::uint8_t {
55 #endif // FLASHLIGHT_FLASHLIGHT_H_
Definition: flashlight.h:31
bool SetBrightness(int brightness, i2c::Error *ec)
Definition: flashlight.cc:99
Mode mode()
Definition: flashlight.h:43
Definition: flashlight.h:24
Flashlight(i2c::Device const &dev)
Definition: flashlight.cc:69
bool Init(i2c::Error *ec)
Definition: flashlight.cc:73
bool SetEnabled(bool enabled, i2c::Error *ec)
Definition: flashlight.cc:143
int brightness()
Definition: flashlight.h:41
bool enabled()
Definition: flashlight.h:42
bool SetMode(Mode mode, i2c::Error *ec)
Definition: flashlight.cc:118
Mode
Definition: flashlight.h:26
int Error
Definition: i2c_new.h:30