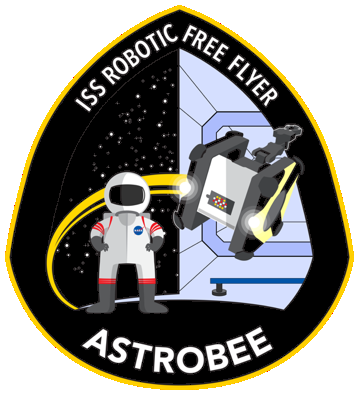 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FF_UTIL_FF_ACTION_H_
20 #define FF_UTIL_FF_ACTION_H_
26 #include <actionlib/server/simple_action_server.h>
27 #include <actionlib/client/simple_action_client.h>
53 template <
class ActionSpec >
76 void Create(ros::NodeHandle *nh, std::string
const& topic) {
77 sas_ = std::shared_ptr < actionlib::SimpleActionServer < ActionSpec > >(
78 new actionlib::SimpleActionServer < ActionSpec > (*nh, topic,
false));
87 sas_->publishFeedback(feedback);
93 switch (result_code) {
95 sas_->setSucceeded(result);
98 sas_->setPreempted(result);
101 sas_->setAborted(result);
112 if (
sas_->isNewGoalAvailable()) {
124 boost::shared_ptr<const Goal> goal =
sas_->acceptNewGoal();
130 std::shared_ptr < actionlib::SimpleActionServer < ActionSpec > >
sas_;
153 template <
class ActionSpec >
161 WAITING_FOR_RESPONSE,
164 static constexpr
double DEFAULT_TIMEOUT_CONNECTED = 0.0;
165 static constexpr
double DEFAULT_TIMEOUT_ACTIVE = 0.0;
166 static constexpr
double DEFAULT_TIMEOUT_RESPONSE = 0.0;
167 static constexpr
double DEFAULT_TIMEOUT_DEADLINE = 0.0;
168 static constexpr
double DEFAULT_POLL_DURATION = 0.1;
169 static constexpr
double DEFAULT_TIMEOUT_RESPONSE_DELAY = 0.1;
207 bool Create(ros::NodeHandle *nh, std::string
const& topic) {
222 sac_ = std::shared_ptr < actionlib::SimpleActionClient < ActionSpec > > (
223 new actionlib::SimpleActionClient < ActionSpec > (*nh, topic,
false));
225 state_ = WAITING_FOR_GOAL;
229 state_ = WAITING_FOR_CONNECT;
235 return sac_->isServerConnected();
240 return (
sac_ &&
sac_->isServerConnected());
245 if (!
sac_)
return false;
255 state_ = WAITING_FOR_ACTIVE;
262 if (!
sac_)
return false;
265 case WAITING_FOR_ACTIVE:
266 case WAITING_FOR_RESPONSE:
267 case WAITING_FOR_DEADLINE:
270 sac_->stopTrackingGoal();
271 state_ = WAITING_FOR_GOAL;
282 if (duration.isZero())
return;
284 timer.setPeriod(duration);
304 state_ = WAITING_FOR_GOAL;
309 if (!
sac_ || !
sac_->isServerConnected())
return;
312 state_ = WAITING_FOR_GOAL;
324 ROS_WARN(
"Freeflyer action timed out on going active.");
350 state_ = WAITING_FOR_RESPONSE;
364 void ResultCallback(actionlib::SimpleClientGoalState
const& action_state, ResultConstPtr
const& result) {
367 sac_->stopTrackingGoal();
372 if (action_state == actionlib::SimpleClientGoalState::SUCCEEDED)
374 else if (action_state == actionlib::SimpleClientGoalState::PREEMPTED)
393 state_ = WAITING_FOR_GOAL;
404 std::shared_ptr < actionlib::SimpleActionClient < ActionSpec > >
sac_;
422 #endif // FF_UTIL_FF_ACTION_H_
std::function< void(void) > ConnectedCallbackType
Definition: ff_action.h:178
Definition: ff_action.h:54
ros::Timer timer_active_
Definition: ff_action.h:410
ACTION_DEFINITION(ActionSpec)
void SetActiveCallback(ActiveCallbackType cb_active)
Definition: ff_action.h:197
ros::Timer timer_response_
Definition: ff_action.h:411
void SetPreemptCallback(PreemptCallbackType cb_preempt)
Definition: ff_action.h:72
bool CancelGoal()
Definition: ff_action.h:261
ACTION_DEFINITION(ActionSpec)
bool SendGoal(Goal const &goal)
Definition: ff_action.h:244
void ResponseTimeoutCallback(ros::TimerEvent const &event)
Definition: ff_action.h:336
ros::Duration to_response_delay_
Definition: ff_action.h:403
void SendFeedback(Feedback const &feedback)
Definition: ff_action.h:85
ros::Duration to_connected_
Definition: ff_action.h:398
void ConnectedTimeoutCallback(ros::TimerEvent const &event)
Definition: ff_action.h:318
@ PREEMPTED
Definition: ff_action.h:43
GoalCallbackType cb_goal_
Definition: ff_action.h:131
void ActiveTimeoutCallback(ros::TimerEvent const &event)
Definition: ff_action.h:323
void SetPollTime(double to_poll)
Definition: ff_action.h:204
FreeFlyerActionServer()
Definition: ff_action.h:65
void ResultDelayCallback(ros::TimerEvent const &event)
Definition: ff_action.h:388
ConnectedCallbackType cb_connected_
Definition: ff_action.h:407
@ TIMEOUT_ON_ACTIVE
Definition: ff_action.h:46
A wrapper class around the simple action client.
Definition: ff_action.h:154
ros::Duration to_deadline_
Definition: ff_action.h:401
ros::Duration to_response_
Definition: ff_action.h:400
ResultCallbackType cb_result_
Definition: ff_action.h:406
void ResultCallback(actionlib::SimpleClientGoalState const &action_state, ResultConstPtr const &result)
Definition: ff_action.h:364
~FreeFlyerActionClient()
Definition: ff_action.h:191
void SetDeadlineTimeout(double to_deadline)
Definition: ff_action.h:203
void StopAllTimers()
Definition: ff_action.h:289
@ TIMEOUT_ON_CONNECT
Definition: ff_action.h:45
void SetActiveTimeout(double to_active)
Definition: ff_action.h:201
void SetResultCallback(ResultCallbackType cb_result)
Definition: ff_action.h:195
ros::Timer timer_connected_
Definition: ff_action.h:409
Definition: ff_action.h:39
void SetGoalCallback(GoalCallbackType cb_goal)
Definition: ff_action.h:71
void ActiveCallback()
Definition: ff_action.h:342
std::shared_ptr< actionlib::SimpleActionServer< ActionSpec > > sas_
Definition: ff_action.h:130
~FreeFlyerActionServer()
Definition: ff_action.h:68
FeedbackCallbackType cb_feedback_
Definition: ff_action.h:405
void GoalCallback()
Definition: ff_action.h:123
State state_
Definition: ff_action.h:397
void FeedbackCallback(FeedbackConstPtr const &feedback)
Definition: ff_action.h:354
ros::Duration to_active_
Definition: ff_action.h:399
CancelCallbackType cb_cancel_
Definition: ff_action.h:133
std::function< void(FeedbackConstPtr const &) > FeedbackCallbackType
Definition: ff_action.h:176
ResultConstPtr result_
Definition: ff_action.h:417
void Create(ros::NodeHandle *nh, std::string const &topic)
Definition: ff_action.h:76
ros::Timer timer_response_delay_
Definition: ff_action.h:414
@ ABORTED
Definition: ff_action.h:44
void PreemptCallback()
Definition: ff_action.h:111
std::shared_ptr< actionlib::SimpleActionClient< ActionSpec > > sac_
Definition: ff_action.h:404
void Complete(FreeFlyerActionState::Enum state, ResultConstPtr const &result)
Definition: ff_action.h:297
FreeFlyerActionState::Enum state_response_
Definition: ff_action.h:416
Definition: partition_image_sequences.cc:48
std::function< void(void) > CancelCallbackType
Definition: ff_action.h:62
ros::Timer timer_deadline_
Definition: ff_action.h:412
Enum
Definition: ff_action.h:41
void ConnectPollCallback(ros::TimerEvent const &event)
Definition: ff_action.h:308
bool Create(ros::NodeHandle *nh, std::string const &topic)
Definition: ff_action.h:207
ActiveCallbackType cb_active_
Definition: ff_action.h:408
FreeFlyerActionClient()
Definition: ff_action.h:182
void SetFeedbackCallback(FeedbackCallbackType cb_feedback)
Definition: ff_action.h:194
bool IsConnected()
Definition: ff_action.h:239
void StartOptionalTimer(ros::Timer &timer, ros::Duration const &duration)
Definition: ff_action.h:281
std::function< void(void) > ActiveCallbackType
Definition: ff_action.h:179
void SetCancelCallback(CancelCallbackType cb_cancel)
Definition: ff_action.h:73
@ SUCCESS
Definition: ff_action.h:42
@ TIMEOUT_ON_DEADLINE
Definition: ff_action.h:48
Definition: config_client.h:31
uint8_t state
Definition: signal_lights.h:90
PreemptCallbackType cb_preempt_
Definition: ff_action.h:132
@ TIMEOUT_ON_RESPONSE
Definition: ff_action.h:47
void DeadlineTimeoutCallback(ros::TimerEvent const &event)
Definition: ff_action.h:330
void SetResponseTimeout(double to_response)
Definition: ff_action.h:202
void SendResult(FreeFlyerActionState::Enum result_code, Result const &result)
Definition: ff_action.h:91
ros::Duration to_poll_
Definition: ff_action.h:402
ros::Timer timer_poll_
Definition: ff_action.h:413
std::function< void(void) > PreemptCallbackType
Definition: ff_action.h:61
void SetConnectedCallback(ConnectedCallbackType cb_connected)
Definition: ff_action.h:196
std::function< void(GoalConstPtr const &) > GoalCallbackType
Definition: ff_action.h:60
void SetConnectedTimeout(double to_connected)
Definition: ff_action.h:200
std::function< void(FreeFlyerActionState::Enum, ResultConstPtr const &) > ResultCallbackType
Definition: ff_action.h:177