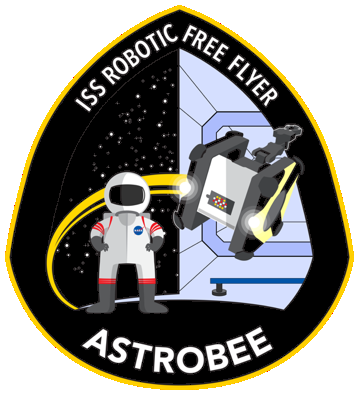 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef COMMS_BRIDGE_BRIDGE_PUBLISHER_H_
20 #define COMMS_BRIDGE_BRIDGE_PUBLISHER_H_
30 #include <topic_tools/shape_shifter.h>
38 #include <condition_variable>
45 typedef std::chrono::time_point<std::chrono::steady_clock>
timepoint_t;
93 std::queue<ContentInfo>
138 #endif // COMMS_BRIDGE_BRIDGE_PUBLISHER_H_
std::shared_ptr< ros::Subscriber > SubscriberPtr
Definition: bridge_publisher.h:43
Definition: bridge_publisher.h:47
unsigned int relay_seqnum
Definition: bridge_publisher.h:87
void drainWaitingQueue(RelayTopicInfo &topic_info)
Definition: bridge_publisher.cpp:227
Definition: bridge_publisher.h:71
timepoint_t delay_until
Definition: bridge_publisher.h:95
std::string type_md5_sum
Definition: bridge_publisher.h:74
unsigned int m_verbose_
Definition: bridge_publisher.h:125
bool relayMessage(RelayTopicInfo &topic_info, const ContentInfo &content_info)
Definition: bridge_publisher.cpp:146
virtual ~BridgePublisher()
Definition: bridge_publisher.cpp:45
std::mutex m_mutex_
Definition: bridge_publisher.h:129
Definition: bridge_publisher.h:56
AdvertisementInfo ad_info
Definition: bridge_publisher.h:91
BridgePublisher(double ad2pub_delay)
Definition: bridge_publisher.cpp:38
std::shared_ptr< std::thread > worker_thread_
Definition: bridge_publisher.h:130
std::string data_type
Definition: bridge_publisher.h:62
unsigned int m_n_relayed_
Definition: bridge_publisher.h:135
std::condition_variable m_drain_cv_
Definition: bridge_publisher.h:133
std::queue< ContentInfo > waiting_msgs
Definition: bridge_publisher.h:94
std::shared_ptr< ros::Publisher > PublisherPtr
Definition: bridge_publisher.h:44
double m_ad2pub_delay_
Definition: bridge_publisher.h:127
bool latching
Definition: bridge_publisher.h:59
Definition: bridge_publisher.h:80
std::string out_topic
Definition: bridge_publisher.h:85
bool advertised
Definition: bridge_publisher.h:86
RelayTopicInfo()
Definition: bridge_publisher.h:82
std::priority_queue< std::pair< timepoint_t, std::string > > m_drain_queue_
Definition: bridge_publisher.h:132
bool advertiseTopic(const std::string &output_topic, const AdvertisementInfo &ad_info)
Definition: bridge_publisher.cpp:51
bool relayContent(RelayTopicInfo &topic_info, const ContentInfo &content_info)
Definition: bridge_publisher.cpp:107
void drainThread()
Definition: bridge_publisher.cpp:194
std::chrono::time_point< std::chrono::steady_clock > timepoint_t
Definition: bridge_publisher.h:45
ros::Publisher publisher
Definition: bridge_publisher.h:84
std::map< std::string, RelayTopicInfo > m_relay_topics_
Definition: bridge_publisher.h:131
std::vector< uint8_t > data
Definition: bridge_publisher.h:77
void setVerbosity(unsigned int verbosity)
Definition: bridge_publisher.cpp:49
std::string md5_sum
Definition: bridge_publisher.h:65
std::string definition
Definition: bridge_publisher.h:68