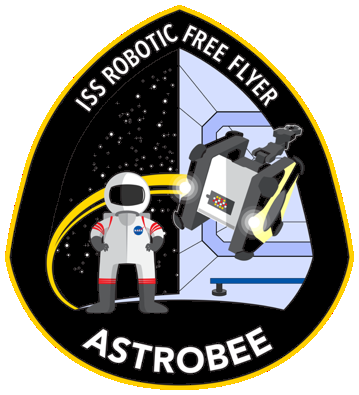 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef CUSTOM_I2C_I2C_H_
20 #define CUSTOM_I2C_I2C_H_
24 #endif // CUSTOM_I2C_I2C_H_
31 int i2c_open(
const char* bus, uint8_t addr);
40 int i2c_write(
int handle, uint8_t* buf,
unsigned int length);
41 int i2c_read(
int handle, uint8_t* buf,
unsigned int length);
43 unsigned int len_w, uint8_t addr_r, uint8_t* buf_r,
int i2c_write(int handle, uint8_t *buf, unsigned int length)
Definition: i2c.cc:57
int i2c_read_no_ack(int handle, uint8_t addr_r, uint8_t *buf, unsigned int length)
Definition: i2c.cc:148
int i2c_read_byte(int handle, uint8_t *val)
Definition: i2c.cc:82
int i2c_set_timeout(int handle, int timeout)
Definition: i2c.cc:38
int i2c_write_ignore_nack(int handle, uint8_t addr_w, uint8_t *buf, unsigned int length)
Definition: i2c.cc:128
int i2c_close(int handle)
Definition: i2c.cc:91
int delay_ms(unsigned int msec)
Definition: i2c.cc:168
int i2c_write_byte(int handle, uint8_t val)
Definition: i2c.cc:65
int i2c_read(int handle, uint8_t *buf, unsigned int length)
Definition: i2c.cc:74
int i2c_open(const char *bus, uint8_t addr)
Definition: i2c.cc:42
int i2c_set_retries(int handle, int retries)
Definition: i2c.cc:34
int i2c_write_read(int handle, uint8_t addr_w, uint8_t *buf_w, unsigned int len_w, uint8_t addr_r, uint8_t *buf_r, unsigned int len_r)
Definition: i2c.cc:101