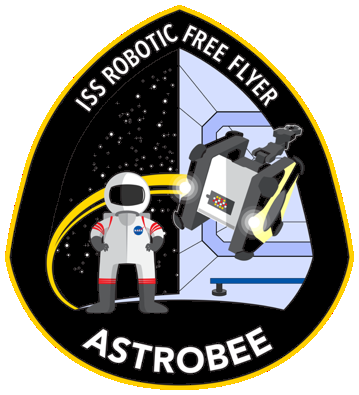 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FF_UTIL_CONFIG_CLIENT_H_
20 #define FF_UTIL_CONFIG_CLIENT_H_
26 #include <dynamic_reconfigure/Reconfigure.h>
35 explicit ConfigClient(ros::NodeHandle *platform_nh,
const std::string &node);
40 template<
typename T>
bool Set(
const std::string &
name,
const T &value);
41 template<
typename T>
bool Get(
const std::string &
name, T &value);
42 template<
typename T> T
Get(
const std::string &
name) {
45 ROS_ERROR_STREAM(
"Cannot query parameter " <<
name);
52 ros::ServiceClient service_;
53 dynamic_reconfigure::ReconfigureRequest request_;
56 template<>
bool ConfigClient::Get<int>(
const std::string &
name,
int &value);
57 template<>
bool ConfigClient::Get<double>(
const std::string &
name,
double &value);
58 template<>
bool ConfigClient::Get<bool>(
const std::string &
name,
bool &value);
59 template<>
bool ConfigClient::Get<std::string>(
const std::string &
name, std::string &value);
61 template<>
bool ConfigClient::Set<int>(
const std::string &
name,
const int &value);
62 template<>
bool ConfigClient::Set<double>(
const std::string &
name,
const double &value);
63 template<>
bool ConfigClient::Set<bool>(
const std::string &
name,
const bool &value);
64 template<>
bool ConfigClient::Set<std::string>(
const std::string &
name,
const std::string &value);
68 #endif // FF_UTIL_CONFIG_CLIENT_H_
virtual ~ConfigClient()
Definition: config_client.cc:38
bool Reconfigure()
Definition: config_client.cc:137
Definition: config_client.h:32
T Get(const std::string &name)
Definition: config_client.h:42
bool Set(const std::string &name, const T &value)
Definition: config_client.cc:49
std::string name
Definition: eps_simulator.cc:48
ConfigClient(ros::NodeHandle *platform_nh, const std::string &node)
Definition: config_client.cc:33
bool Get(const std::string &name, T &value)
Definition: config_client.cc:43
Definition: config_client.h:31