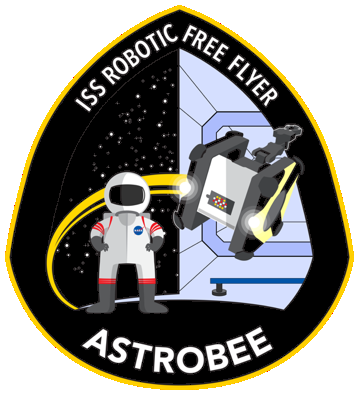 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
22 #ifndef CONFIG_READER_WATCH_FILES_H_
23 #define CONFIG_READER_WATCH_FILES_H_
28 #include <sys/inotify.h>
30 #include <sys/types.h>
50 void init(
const char *_name)
53 {
return(
name.c_str());}
65 static const uint32_t
ModEvents = IN_CLOSE_WRITE|IN_DELETE_SELF;
81 {
return(_parent && _parent->
addWatch(
this, filename));}
109 bool addWatch(Watch *w,
const char *filename);
125 #endif // CONFIG_READER_WATCH_FILES_H_
Definition: config_reader.h:46
bool addWatch(Watch *w, const char *filename)
Definition: watch_files.cc:75
time_t mod_time
Definition: watch_files.h:43
void SetNonBlocking(int fd)
Definition: watch_files.cc:42
~WatchFiles()
Definition: watch_files.h:101
uint32_t calcEventMask(Watch *w)
Definition: watch_files.cc:102
WatchFiles * parent
Definition: watch_files.h:70
time_t getFileModTime()
Definition: watch_files.cc:50
bool rewatch(const char *filename)
Definition: watch_files.h:82
std::string name
Definition: watch_files.h:41
WatchFiles()
Definition: watch_files.h:99
const char * operator()()
Definition: watch_files.h:52
Definition: watch_files.h:63
int inotify_fd
Definition: watch_files.h:95
void init(const char *_name)
Definition: watch_files.h:50
bool valid()
Definition: watch_files.h:78
void reset()
Definition: watch_files.cc:66
time_t FileModTime(const char *filename)
Definition: watch_files.cc:35
static const uint32_t ModEvents
Definition: watch_files.h:65
void clearEvents()
Definition: watch_files.h:116
Watch(const Watch &w)
Definition: watch_files.h:75
int num_watches
Definition: watch_files.h:96
bool watch(WatchFiles *_parent, const char *filename)
Definition: watch_files.h:80
Definition: watch_files.h:68
bool init()
Definition: watch_files.cc:56
bool isFileModified()
Definition: watch_files.h:87
int getEvents()
Definition: watch_files.cc:110
bool isInited()
Definition: watch_files.h:106
int getNumEvents()
Definition: watch_files.h:114
std::vector< inotify_event > events
Definition: watch_files.h:94
Definition: watch_files.h:39
Watch()
Definition: watch_files.h:73
ActiveFile()
Definition: watch_files.h:47
void markAsRead()
Definition: watch_files.h:59
bool removeWatch(Watch *w)
Definition: watch_files.cc:91
int wd
Definition: watch_files.h:71
bool isModified()
Definition: watch_files.h:57
bool remove()
Definition: watch_files.h:84
void invalidate()
Definition: watch_files.h:55