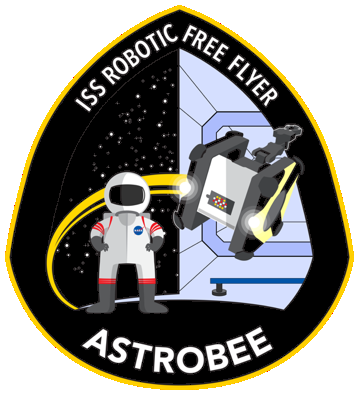 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_PRO_TRAJECTORY_SOLVER_H_
20 #define TRAJ_OPT_PRO_TRAJECTORY_SOLVER_H_
24 #include <boost/smart_ptr/shared_ptr.hpp>
50 const std::vector<Waypoint> &waypnts,
const std::vector<MatD> &A,
51 const std::vector<VecD> &b,
const std::vector<decimal_t> &ds,
53 boost::shared_ptr<Vec3Vec> points = boost::shared_ptr<Vec3Vec>(),
68 boost::shared_ptr<Trajectory>
traj_;
75 #endif // TRAJ_OPT_PRO_TRAJECTORY_SOLVER_H_
VecD vel
Definition: trajectory_solver.h:34
bool use_acc
Definition: trajectory_solver.h:39
virtual bool adjustTimes(decimal_t epsilon)
Definition: trajectory_solver.cpp:39
virtual bool solveTrajectory(const std::vector< Waypoint > &waypnts, const std::vector< MatD > &A, const std::vector< VecD > &b, const std::vector< decimal_t > &ds, decimal_t epsilon=0, boost::shared_ptr< Vec3Vec > points=boost::shared_ptr< Vec3Vec >(), decimal_t upsilon=0)
Definition: trajectory_solver.cpp:27
VecD jrk
Definition: trajectory_solver.h:36
decimal_t j_max_
Definition: trajectory_solver.h:71
bool trajectory_solved_
Definition: trajectory_solver.h:67
bool use_pos
Definition: trajectory_solver.h:37
virtual void setParams(decimal_t v_max, decimal_t a_max, decimal_t j_max, int time_its, decimal_t time_eps)
Definition: trajectory_solver.cpp:41
boost::shared_ptr< Trajectory > traj_
Definition: trajectory_solver.h:68
int time_its_
Definition: trajectory_solver.h:72
Definition: trajectory_solver.h:31
double decimal_t
Definition: types.h:35
virtual boost::shared_ptr< Trajectory > getTrajectory()
Definition: trajectory_solver.cpp:36
decimal_t time_eps_
Definition: trajectory_solver.h:71
int knot_id
Definition: trajectory_solver.h:32
VecD acc
Definition: trajectory_solver.h:35
std::pair< Eigen::VectorXi, MatD > getIndexForm() const
Definition: trajectory_solver.cpp:51
Definition: msg_traj.h:27
virtual bool checkMax(decimal_t r)
Definition: trajectory_solver.h:63
TrajectorySolver()
Definition: trajectory_solver.cpp:25
std::vector< Waypoint > waypoints_
Definition: trajectory_solver.h:66
decimal_t a_max_
Definition: trajectory_solver.h:71
Definition: trajectory_solver.h:46
virtual bool trajectoryStatus()
Definition: trajectory_solver.cpp:35
bool use_vel
Definition: trajectory_solver.h:38
bool use_jrk
Definition: trajectory_solver.h:40
VecD pos
Definition: trajectory_solver.h:33
decimal_t v_max_
Definition: trajectory_solver.h:71
Eigen::Matrix< decimal_t, Eigen::Dynamic, 1 > VecD
Definition: types.h:49