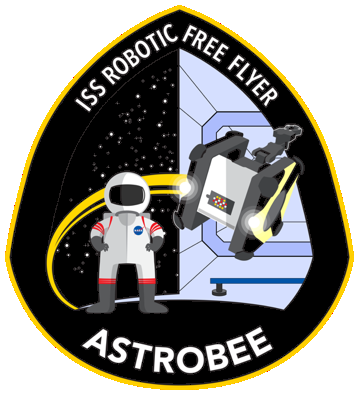 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef NODES_TIMESTAMPED_NODES_H_
19 #define NODES_TIMESTAMPED_NODES_H_
24 template <
typename NodeType>
38 gtsam::KeyVector AddNode(
const NodeType& node)
final;
40 boost::optional<NodeType> GetNode(
const gtsam::KeyVector& keys,
45 template <
class Archive>
46 void serialize(Archive& ar,
const unsigned int file_version) {
47 ar& BOOST_SERIALIZATION_BASE_OBJECT_NVP(
Base);
52 template <
typename NodeType>
56 template <
typename NodeType>
58 const auto key = this->values_->
Add(node);
62 template <
typename NodeType>
63 boost::optional<NodeType> TimestampedNodes<NodeType>::GetNode(
const gtsam::KeyVector& keys,
70 #endif // NODES_TIMESTAMPED_NODES_H_
TimestampedNodes()=default
Definition: combined_nav_state_nodes.h:24
friend class boost::serialization::access
Definition: timestamped_nodes.h:44
Definition: timestamped_combined_nodes.h:44
gtsam::KeyVector Add(const localization_common::Time timestamp, const NodeType &node)
Definition: timestamped_combined_nodes.h:174
Definition: timestamped_nodes.h:28
double Time
Definition: time.h:23