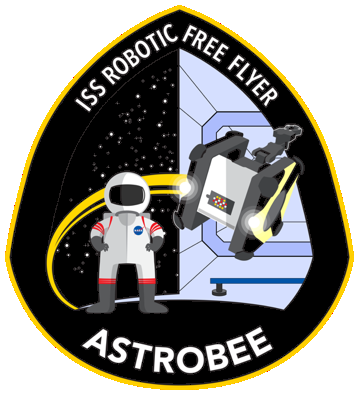 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef LOCALIZATION_COMMON_STATS_LOGGER_H_
19 #define LOCALIZATION_COMMON_STATS_LOGGER_H_
32 explicit StatsLogger(
const bool log_on_destruction =
true);
46 void LogToFile(std::ofstream& ofstream)
const;
49 void LogToCsv(std::ofstream& ofstream)
const;
51 std::vector<std::reference_wrapper<localization_common::Timer>>
timers_;
52 std::vector<std::reference_wrapper<localization_common::Averager>>
averagers_;
55 template <
typename Logger>
56 void LogToFile(
const std::vector<std::reference_wrapper<Logger>>& loggers, std::ofstream& ofstream)
const {
57 for (
const auto& logger : loggers) logger.get().LogToFile(ofstream);
60 template <
typename Logger>
61 void LogToCsv(
const std::vector<std::reference_wrapper<Logger>>& loggers, std::ofstream& ofstream)
const {
62 for (
const auto& logger : loggers) logger.get().LogToCsv(ofstream);
65 template <
typename Logger>
66 void Log(
const std::vector<std::reference_wrapper<Logger>>& loggers)
const {
67 for (
const auto& logger : loggers) logger.get().Log();
70 bool log_on_destruction_;
74 #endif // LOCALIZATION_COMMON_STATS_LOGGER_H_
Definition: averager.h:33
~StatsLogger()
Definition: stats_logger.cc:23
void AddTimer(localization_common::Timer &timer)
Definition: stats_logger.cc:29
std::vector< std::reference_wrapper< localization_common::Timer > > timers_
Definition: stats_logger.h:51
Definition: averager.h:34
void AddAverager(localization_common::Averager &averager)
Definition: stats_logger.cc:27
Definition: stats_logger.h:30
std::vector< std::reference_wrapper< localization_common::Averager > > averagers_
Definition: stats_logger.h:52
void Log() const
Definition: stats_logger.cc:31
void LogToFile(std::ofstream &ofstream) const
Definition: stats_logger.cc:36
StatsLogger(const bool log_on_destruction=true)
Definition: stats_logger.cc:21
void LogToCsv(std::ofstream &ofstream) const
Definition: stats_logger.cc:41