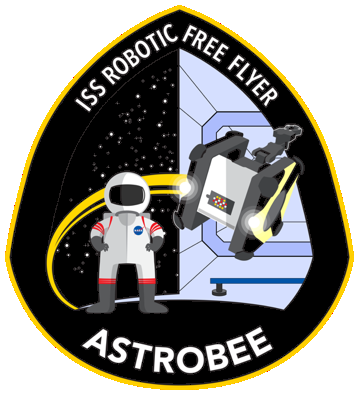 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef LOCALIZATION_RVIZ_PLUGINS_SLIDER_PROPERTY_H // NOLINT
19 #define LOCALIZATION_RVIZ_PLUGINS_SLIDER_PROPERTY_H // NOLINT
21 #include <rviz/properties/property.h>
29 SliderProperty(
const QString&
name = QString(),
const int default_value = 0,
const QString& description = QString(),
30 Property* parent =
nullptr,
const char* changed_slot =
nullptr, QObject* receiver =
nullptr);
32 QWidget*
createEditor(QWidget* parent,
const QStyleOptionViewItem& option)
override;
54 #endif // LOCALIZATION_RVIZ_PLUGINS_SLIDER_PROPERTY_H // NOLINT
QSlider * slider_
Definition: slider_property.h:46
int min_
Definition: slider_property.h:48
QWidget * createEditor(QWidget *parent, const QStyleOptionViewItem &option) override
Definition: slider_property.cc:25
int value_
Definition: slider_property.h:49
std::string name
Definition: eps_simulator.cc:48
Definition: trajectory_display.h:32
int getInt() const
Definition: slider_property.cc:39
Definition: slider_property.h:26
void setValue(const int value)
Definition: slider_property.cc:33
void setMinimum(const int min)
Definition: slider_property.cc:37
SliderProperty(const QString &name=QString(), const int default_value=0, const QString &description=QString(), Property *parent=nullptr, const char *changed_slot=nullptr, QObject *receiver=nullptr)
Definition: slider_property.cc:21
void setMaximum(const int max)
Definition: slider_property.cc:35
int max_
Definition: slider_property.h:47