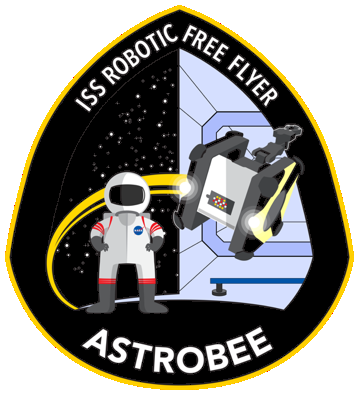 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef LOCALIZATION_COMMON_AVERAGER_H_
20 #define LOCALIZATION_COMMON_AVERAGER_H_
22 #include <boost/accumulators/accumulators.hpp>
23 #include <boost/accumulators/statistics/count.hpp>
24 #include <boost/accumulators/statistics/max.hpp>
25 #include <boost/accumulators/statistics/mean.hpp>
26 #include <boost/accumulators/statistics/min.hpp>
27 #include <boost/accumulators/statistics/stats.hpp>
28 #include <boost/accumulators/statistics/variance.hpp>
36 explicit Averager(
const std::string& averager_name =
"",
const std::string& type_name =
"",
37 const std::string& units =
"",
const bool log_on_destruction =
false);
39 void Update(
const double value);
44 void LogToFile(std::ofstream& ofstream)
const;
45 void LogToCsv(std::ofstream& ofstream)
const;
46 void LogEveryN(
const int num_events_per_log)
const;
49 void Vlog(
const int level = 2)
const;
50 void VlogEveryN(
const int num_events_per_log,
const int level)
const;
53 std::string StatsString()
const;
54 std::string CsvStatsString()
const;
55 std::string LastValueString()
const;
58 std::string type_name_;
62 bool log_on_destruction_;
63 boost::accumulators::accumulator_set<
64 double, boost::accumulators::stats<boost::accumulators::tag::mean(boost::accumulators::immediate),
65 boost::accumulators::tag::variance(boost::accumulators::immediate),
66 boost::accumulators::tag::min, boost::accumulators::tag::max,
67 boost::accumulators::tag::count>>
72 #endif // LOCALIZATION_COMMON_AVERAGER_H_
Definition: averager.h:33
void UpdateAndLogEveryN(const double value, const int num_events_per_log)
Definition: averager.cc:84
void LogToCsv(std::ofstream &ofstream) const
Definition: averager.cc:77
~Averager()
Definition: averager.cc:30
Definition: averager.h:34
void Update(const double value)
Definition: averager.cc:34
void VlogEveryN(const int num_events_per_log, const int level) const
Definition: averager.cc:100
void UpdateAndLog(const double value)
Definition: averager.cc:79
void LogEveryN(const int num_events_per_log) const
Definition: averager.cc:94
void Log() const
Definition: averager.cc:70
Averager(const std::string &averager_name="", const std::string &type_name="", const std::string &units="", const bool log_on_destruction=false)
Definition: averager.cc:23
int count() const
Definition: averager.cc:41
void LogToFile(std::ofstream &ofstream) const
Definition: averager.cc:75
double last_value() const
Definition: averager.cc:43
void Vlog(const int level=2) const
Definition: averager.cc:89
double average() const
Definition: averager.cc:39