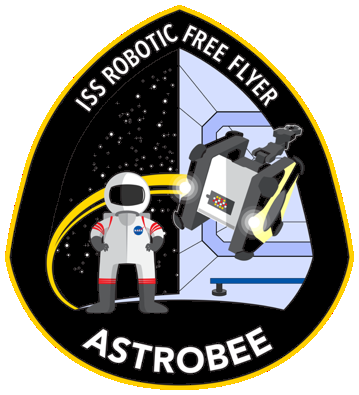 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
22 #ifndef CONFIG_READER_CONFIG_READER_H_
23 #define CONFIG_READER_CONFIG_READER_H_
31 #include <glog/logging.h>
35 #include <sys/types.h>
67 bool GetStr(
const char *exp, std::string *str);
68 bool GetStr(
int index, std::string *str);
69 bool GetBool(
const char *exp,
bool *val);
70 bool GetBool(
int index,
bool *val);
71 bool GetInt(
const char *exp,
int *val);
72 bool GetInt(
int index,
int *val);
75 bool GetUInt(
const char *exp,
unsigned int *val);
76 bool GetUInt(
int index,
unsigned int *val);
77 bool GetReal(
const char *exp,
float *val);
78 bool GetReal(
int index,
float *val);
79 bool GetReal(
const char *exp,
double *val);
80 bool GetReal(
int index,
double *val);
85 bool GetInt(
const char *exp,
int *val,
int min,
int max);
86 bool GetInt(
int index,
int *val,
int min,
int max);
87 bool GetReal(
const char *exp,
float *val,
float min,
float max);
88 bool GetReal(
int index,
float *val,
float min,
float max);
89 bool GetReal(
const char *exp,
double *val,
double min,
double max);
90 bool GetReal(
int index,
double *val,
double min,
double max);
99 std::string full_exp_;
110 void SetPath(
const char* path);
111 void AddFile(
const char *filename,
unsigned flags = 0);
117 bool GetTable(
const char *exp, Table *table);
118 bool GetStr(
const char *exp, std::string *str);
119 bool GetBool(
const char *exp,
bool *val);
120 bool GetInt(
const char *exp,
int *val);
122 bool GetUInt(
const char *exp,
unsigned int *val);
123 bool GetReal(
const char *exp,
float *val);
124 bool GetReal(
const char *exp,
double *val);
126 bool GetPosReal(
const char *exp,
double *val);
127 bool GetInt(
const char *exp,
int *val,
int min,
int max);
128 bool GetReal(
const char *exp,
float *val,
float min,
float max);
129 bool GetReal(
const char *exp,
double *val,
double min,
double max);
145 void ShowError(
int err_code,
const char *filename);
146 bool ReadFile(
const char *filename,
unsigned flags);
155 bool ReadTable(
const char *exp, Table *table);
156 bool ReadStr(
const char *exp, std::string *str);
157 bool ReadBool(
const char *exp,
bool *val);
158 bool ReadInt(
const char *exp,
int *val);
160 bool ReadUInt(
const char *exp,
unsigned int *val);
161 bool ReadReal(
const char *exp,
float *val);
162 bool ReadReal(
const char *exp,
double *val);
165 bool ReadInt(
const char *exp,
int *val,
int min,
int max);
166 bool ReadReal(
const char *exp,
float *val,
float min,
float max);
167 bool ReadReal(
const char *exp,
double *val,
double min,
double max);
181 #endif // CONFIG_READER_CONFIG_READER_H_
bool IsInit()
Definition: config_reader.cc:96
bool GetUInt(const char *exp, unsigned int *val)
Definition: config_reader.cc:248
bool GetUInt(const char *exp, unsigned int *val)
Definition: config_reader.cc:626
~ConfigReader()
Definition: config_reader.cc:490
Definition: config_reader.h:46
void ClearWatches()
Definition: config_reader.cc:717
bool ReadFiles()
Definition: config_reader.cc:530
bool ReadPosReal(const char *exp, float *val)
Definition: config_reader.cc:1019
bool GetTable(const char *exp, Table *table)
Definition: config_reader.cc:586
std::vector< FileHeader > files_
Definition: config_reader.h:170
bool IsNumber(const char *exp)
Definition: config_reader.cc:452
FileFlags
Definition: config_reader.h:50
bool GetPosReal(const char *exp, float *val)
Definition: config_reader.cc:650
bool IsFileModified()
Definition: config_reader.cc:557
void CheckFilesUpdated(std::function< void(void)>)
Definition: config_reader.cc:567
bool PutObjectOnLuaStack(const char *exp)
Definition: config_reader.cc:834
bool GetStr(const char *exp, std::string *str)
Definition: config_reader.cc:594
bool ReadFile(const char *filename, unsigned flags)
Definition: config_reader.cc:747
WatchFiles watch_files_
Definition: config_reader.h:172
Definition: watch_files.h:63
bool IsOpen()
Definition: config_reader.cc:505
void ShowError(int err_code, const char *filename)
Definition: config_reader.cc:723
bool GetPosReal(const char *exp, float *val)
Definition: config_reader.cc:323
void OutputGetValueError(const char *exp, const char *type)
Definition: config_reader.cc:906
bool GetBool(const char *exp, bool *val)
Definition: config_reader.cc:602
bool FileExists(const char *filename)
Definition: config_reader.cc:41
@ Optional
Definition: config_reader.h:51
bool modified_
Definition: config_reader.h:173
bool ReadLongLong(const char *exp, int64_t *val)
Definition: config_reader.cc:967
bool GetTable(const char *exp, Table *table)
Definition: config_reader.cc:123
void AddFile(const char *filename, unsigned flags=0)
Definition: config_reader.cc:517
bool InitLua()
Definition: config_reader.cc:691
bool CheckValExists(const char *exp)
Definition: config_reader.cc:105
bool ReadStr(const char *exp, std::string *str)
Definition: config_reader.cc:931
ConfigReader * Config()
Definition: config_reader.h:92
bool GetLongLong(const char *exp, int64_t *val)
Definition: config_reader.cc:618
int GetSize()
Definition: config_reader.cc:119
Definition: watch_files.h:68
bool GetInt(const char *exp, int *val)
Definition: config_reader.cc:198
bool Init(const ros::NodeHandle &nh)
Definition: fam_cmd_i2c_node.cc:62
Definition: config_reader.h:48
void Reset()
Definition: config_reader.cc:498
std::string path_
Definition: config_reader.h:174
bool ReadUInt(const char *exp, unsigned int *val)
Definition: config_reader.cc:979
bool ReadInt(const char *exp, int *val)
Definition: config_reader.cc:955
bool GetReal(const char *exp, float *val)
Definition: config_reader.cc:273
bool GetStr(const char *exp, std::string *str)
Definition: config_reader.cc:148
bool ReadReal(const char *exp, float *val)
Definition: config_reader.cc:995
bool IsNumber()
Definition: config_reader.cc:1111
bool GetLongLong(const char *exp, int64_t *val)
Definition: config_reader.cc:223
lua_State * l_
Definition: config_reader.h:171
void CloseLua()
Definition: config_reader.cc:710
bool ReadBool(const char *exp, bool *val)
Definition: config_reader.cc:943
Definition: config_reader.h:54
Table()
Definition: config_reader.cc:47
bool GetReal(const char *exp, float *val)
Definition: config_reader.cc:634
ConfigReader()
Definition: config_reader.cc:473
bool IsTopValid()
Definition: config_reader.cc:892
bool GetBool(const char *exp, bool *val)
Definition: config_reader.cc:173
void SetPath(const char *path)
Definition: config_reader.cc:509
bool ReadTable(const char *exp, Table *table)
Definition: config_reader.cc:916
void AddStandard()
Definition: config_reader.cc:829
bool GetInt(const char *exp, int *val)
Definition: config_reader.cc:610
bool CheckValExists(const char *exp)
Definition: config_reader.cc:578
void Close()
Definition: config_reader.cc:494
void AddImports()
Definition: config_reader.cc:770