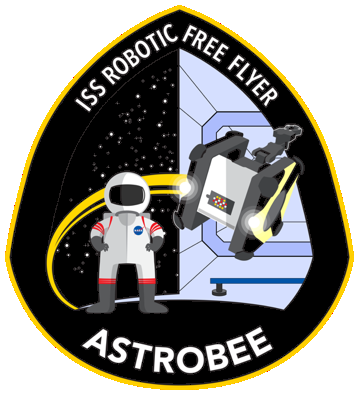 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef VISION_COMMON_SPACED_FEATURE_TRACK_H_
20 #define VISION_COMMON_SPACED_FEATURE_TRACK_H_
48 int MaxSpacing(
const int max_num_points)
const;
54 bool SpacingFits(
const int spacing,
const int max_num_points)
const;
55 int ClosestSpacing(
const int ideal_spacing,
const int ideal_max_num_points)
const;
59 template <
class ARCHIVE>
60 void serialize(ARCHIVE& ar,
const unsigned int ) {
66 #endif // VISION_COMMON_SPACED_FEATURE_TRACK_H_
Definition: brisk_feature_detector_and_matcher.h:25
boost::optional< localization_common::Time > SecondLatestTimestamp() const
Definition: spaced_feature_track.cc:69
std::vector< FeaturePoint > LatestSpacedPoints(const int spacing=0) const
Definition: spaced_feature_track.cc:26
Definition: feature_track.h:28
int MaxSpacing(const int max_num_points) const
Definition: spaced_feature_track.cc:48
Definition: spaced_feature_track.h:32
SpacedFeatureTrack()=default
virtual ~SpacedFeatureTrack()=default
int FeatureId
Definition: feature_point.h:29
friend class boost::serialization::access
Definition: spaced_feature_track.h:58