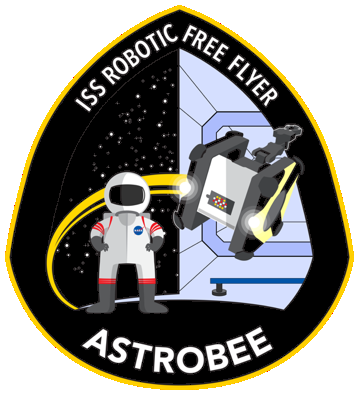 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FF_UTIL_FF_SERVICE_H_
20 #define FF_UTIL_FF_SERVICE_H_
38 template <
class ServiceSpec >
72 bool Create(ros::NodeHandle *nh, std::string
const& topic) {
94 bool Call(ServiceSpec & service) {
103 if (duration.isZero())
return;
105 timer.setPeriod(duration);
152 #endif // FF_UTIL_FF_SERVICE_H_
void SetConnectedCallback(ConnectedCallbackType cb_connected)
Definition: ff_service.h:56
static constexpr double DEFAULT_POLL_DURATION
Definition: ff_service.h:47
std::function< void(void) > ConnectedCallbackType
Definition: ff_service.h:51
ConnectedCallbackType cb_connected_
Definition: ff_service.h:142
Definition: ff_service.h:39
std::string topic_
Definition: ff_service.h:146
void StartOptionalTimer(ros::Timer &timer, ros::Duration const &duration)
Definition: ff_service.h:102
std::function< void(void) > TimeoutCallbackType
Definition: ff_service.h:52
~FreeFlyerServiceClient()
Definition: ff_service.h:67
ros::Timer timer_connected_
Definition: ff_service.h:144
bool IsConnected()
Definition: ff_service.h:86
ros::Duration to_poll_
Definition: ff_service.h:140
void TimeoutCallback(ros::TimerEvent const &event)
Definition: ff_service.h:130
FreeFlyerServiceClient()
Definition: ff_service.h:62
@ WAITING_FOR_CONNECT
Definition: ff_service.h:43
ros::Duration to_connected_
Definition: ff_service.h:139
State
Definition: ff_service.h:41
TimeoutCallbackType cb_timeout_
Definition: ff_service.h:141
void SetConnectedTimeout(double to_connected)
Definition: ff_service.h:59
@ WAITING_FOR_CALL
Definition: ff_service.h:44
@ WAITING_FOR_CREATE
Definition: ff_service.h:42
ros::NodeHandle * nh_
Definition: ff_service.h:147
State state_
Definition: ff_service.h:138
ros::ServiceClient service_
Definition: ff_service.h:143
static constexpr double DEFAULT_TIMEOUT_CONNECTED
Definition: ff_service.h:46
ros::Timer timer_poll_
Definition: ff_service.h:145
void ConnectPollCallback(ros::TimerEvent const &event)
Definition: ff_service.h:110
Definition: config_client.h:31
bool Call(ServiceSpec &service)
Definition: ff_service.h:94
bool Create(ros::NodeHandle *nh, std::string const &topic)
Definition: ff_service.h:72
void SetTimeoutCallback(TimeoutCallbackType cb_timeout)
Definition: ff_service.h:55